It’s very common to copy a list in your Python programs. But, what should you absolutely know about copying lists?
How to copy a Python list?
Python provides multiple ways to copy a list depending on what your program needs to do with the existing list. You can use the assignment operator, the list copy method, the slice notation and shallow or deep copy.
This tutorial is designed to show you everything you need to know about copying lists in Python.
Let’s get started!
How to Make a Copy of a List in Python
I will start with a simple example to understand together how copying list works in Python.
After defining a list called numbers I use the assignment operator ( = ) to copy this list to a new list called new_numbers.
Let’s see what happens…
>>> numbers = [1,4,7,19,23]
>>> new_numbers = numbers
Now I add a new element to the new_numbers list using the append method and verify the elements in both lists using the print function:
>>> new_numbers.append(34)
>>> print(numbers)
[1, 4, 7, 19, 23, 34]
>>> print(new_numbers)
[1, 4, 7, 19, 23, 34]
For some reason even if we have added the new number to the new_numbers list only, both of our lists contain the new number.
Why?
We will use the built-in id function to print the memory address of our two lists and to make it more readable we will also use the hex function that provides an hexadecimal representation of an integer.
>>> hex(id(numbers))
'0x10d75e5a0'
>>> hex(id(new_numbers))
'0x10d75e5a0'
Interesting…
Can you see the problem?
Both variables point to the same memory address, so numbers and new_numbers points to the same list object. That’s why we see the new element in both of them.
So, how can we copy our list to a completely new object?
How to Create An Actual Copy of the Original List
Python provides the list copy method that allows to create a new list object from the one we copy.
Let’s use the copy method on our original list to create the list new_numbers:
new_numbers = numbers.copy()
Now we will append a number to the new list we have created and we will verify that the number is not present in the original list:
>>> new_numbers.append(34)
>>> print(numbers)
[1, 4, 7, 19, 23]
>>> print(new_numbers)
[1, 4, 7, 19, 23, 34]
This time the original list has not been changed by the append method applied to the new list.
And as confirmation we will also verify the memory location of both list objects:
>>> hex(id(numbers))
'0x10751d460'
>>> hex(id(new_numbers))
'0x10761d9b0'
Different memory addresses for the two objects. That’s good!
Copying Using the Python Slice Notation
Another way to copy a Python list is with the slice notation.
The slice notation can be used to copy parts of a list into a new list or even the entire list by simply using the following expression:
new_list = original_list[:]
Let’s apply it to our numbers list:
>>> new_numbers = numbers[:]
After adding another number to the new list you can see that the original list, once again, is unchanged:
>>> new_numbers.append(34)
>>> print(numbers)
[1, 4, 7, 19, 23]
>>> print(new_numbers)
[1, 4, 7, 19, 23, 34]
And that with the slice notation we have created a new list object:
>>> hex(id(numbers))
'0x105e92460'
>>> hex(id(new_numbers))
'0x105f925f0'
And also this one is done! 🙂
Shallow Copy Vs Deep Copy
The difference between a shallow copy and a deep copy only applies to compound objects, in other words to objects that contain other objects.
Examples of compound objects are class instances and lists.
The Python copy module allows to create shallow copies and deep copies of objects. Below you can see the syntax for both types of copy:
SHALLOW COPY: new_object = copy.copy(original_object)
DEEP COPY: new_object = copy.deepcopy(original_object)
With a shallow copy a new compound object is created (e.g. a list of lists) and references to the objects found in the original object are added to the new compound object.
In the next section we will see exactly how a shallow copy works.
In the meantime I want to make clear the difference between a shallow copy and a deep copy.
A deep copy creates a new compound object (e.g. a list of lists) then it also creates copies of the objects found in the original object and inserts them in the new compound object.
The definitions of shallow copy and deep copy will be a lot clearer in the next sections where we will see how they work in practice.
How to Make a Shallow Copy in Python
Let’s see how a shallow copy works with a list…
…try these commands in your Python shell to make sure the behaviour of shallow and deep copying is clear to you:
>>> import copy
>>> numbers = [[1,2,3], [4,5,6], [7,8,9]]
>>> new_numbers = copy.copy(numbers)
If I add an element to the new_numbers list the original list doesn’t change:
>>> new_numbers.append([10,11,12])
>>> numbers
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
>>> new_numbers
[[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]]
This confirms that in the shallow copy a new compound object has been created. In other words the new compound object is not a reference to the original object.
But now, let’s try to update one element common between the original and the new list:
>>> new_numbers[0][0] = 4
>>> numbers
[[4, 2, 3], [4, 5, 6], [7, 8, 9]]
>>> new_numbers
[[4, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]]
I have updated the first element of the first list object in the original list.
As you can see the element has been updated in both lists, the original and the new one.
That’s because we have used a shallow copy and hence the first element of the new_numbers list is just a reference to the first element of the numbers list ([1,2,3]).
How to Make a Deep Copy in Python
Let’s create a deep copy from the same list of lists in the previous section…
>>> import copy
>>> numbers = [[1,2,3], [4,5,6], [7,8,9]]
>>> new_numbers = copy.deepcopy(numbers)
Once again let’s add an element to the new_numbers list:
>>> new_numbers.append([10,11,12])
>>> numbers
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
>>> new_numbers
[[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]]
As expected the original list has not changed after adding a new list to the new list.
In the same way we have done in the previous section, let’s change the first element of the first list in the new_numbers list and see what happens…
>>> new_numbers[0][0] = 4
>>> numbers
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
>>> new_numbers
[[4, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]]
This time, based on the definition of deep copy, the original list has not been changed when we have updated the value of the first element in the first list of the new_numbers list.
Now can you see the difference between a shallow copy and a deep copy?
Conclusion
In this tutorial you have learned how to copy a Python list in multiple different ways:
- Using the assignment operator in the same way you would usually do when assigning a value to a variable.
- With the list copy method.
- Using the Python slice notation [:].
- With a shallow copy or deep copy depending on how you want your compound object to be constructed.
And now you also know how to avoid mistakes caused by the way Python handles the copy of mutable collections like lists 🙂
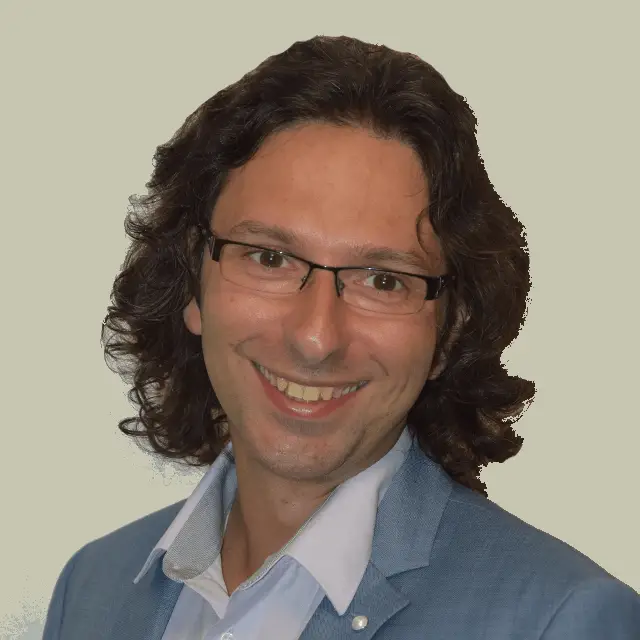
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.