Are you looking at browser automation and are you wondering how to open a web browser with Python? In this tutorial, I will show you how to do it.
To open a web browser Python provides the webbrowser module. You can use the webbrowser module to open the default browser on your system using the webbrowser.open() function. You can select a specific browser to open using the function webbrowser.get().
After going through the basics on how to open a browser we will also build a simple program that will help you quickly set up your environment with a list of websites of your choice.
Let’s start opening browsers! 🙂
Can Python Interact with a Web Browser?
Yes, it can!
Python can interact with a web browser using the webbrowser module.
How can you open a URL in a browser using a Python script?
You can open a URL in the default browser on your machine following the steps below:
- Import the webbrowser Python module.
- Call the open() function of the webbrowser module and pass to it the URL of the website you want to open.
Here is an example…
I have created a Python script called web_browser_test.py and added the following lines of code:
import webbrowser
url = 'https://codefather.tech/blog/'
webbrowser.open(url)
First we import the webbrowser module and we use the string variable url to specify the website we want to open.
Then we call the function webbrowser.open() to open that webpage on our default browser.
Let’s see what happens when I execute this script:
$ python web_browser_test.py
When I execute this script on my machine it opens the CodeFatherTech website in a new Chrome tab.
Before continuing this tutorial try it on your machine and see what happens.
Also according to the official Python documentation the open() function supports an additional argument called new:
webbrowser.open(url, new=0)
In the syntax above new=0 means that the default value for the new argument is zero.
Here is the meaning of the different values for the new argument:
- 0: the url is opened in the same browser window.
- 1: a new browser window is opened.
- 2: a new browser tab is opened.
For the three values of the new argument the Python documentation says that the each action will happen “if possible“.
It’s not very clear what “if possible” means and from a few tests I have done the behaviour of the open() function doesn’t change so clearly depending on the value of the new argument.
Generally the simplest approach is just to use the open() function with its default setting (without specifying the new argument).
How To Open a New Browser Tab in Python
If you want to make your code more explicit and actually make it clear that you want to open a new tab you can use the webbrowser.open_new_tab() function.
You can see that this function is available when you import the webbrowser module:
>>> import webbrowser
>>> dir(webbrowser)
['BackgroundBrowser', 'BaseBrowser', 'Chrome', 'Chromium', 'Elinks', 'Error', 'Galeon', 'GenericBrowser', 'Grail', 'Konqueror', 'MacOSX', 'MacOSXOSAScript', 'Mozilla', 'Netscape', 'Opera', 'UnixBrowser', '__all__', '__builtins__', '__cached__', '__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__', '_browsers', '_lock', '_os_preferred_browser', '_synthesize', '_tryorder', 'get', 'main', 'open', 'open_new', 'open_new_tab', 'os', 'register', 'register_X_browsers', 'register_standard_browsers', 'shlex', 'shutil', 'subprocess', 'sys', 'threading']
Let’s try to call this function!
import webbrowser
url = 'https://codefather.tech/blog/'
webbrowser.open_new_tab(url)
At the moment the default browser on my machine is Chrome where I already have a few tabs open.
When I execute the script a new tab gets opened in Chrome.
Try it on your machine and see what happens.
How to Open a New Browser Page Using Python
What if you want to open a website in a new browser window?
From the output of the dir() built-in function in the previous section you can see that the webbrowser module implements also the function open_new().
The webbrowser module provides the open_new() function that allows to open a URL in a new browser window in Python.
Here is what the official Python documentation says about this function.

Let’s update our script to use this function and see what happens.
import webbrowser
url = 'https://codefather.tech/blog/'
webbrowser.open_new(url)
At the moment I have a Chrome window open with other tabs already open and when I execute this code Python opens a new tab in the existing window.
This is probably the behaviour the Python documentation refers to when it says “otherwise, open url in the only browser window“.
I will change the default browser on my system to find out what happens if I try to open a default browser that is not already open.
On a Mac I can do it going to > System Preferences, then click General and then by updating the browser in the section “Default web browser“.
I have set Safari as my default browser.
Note: these steps will change if you have a different operating system.

Let’s execute the Python script again…
$ python web_browser_test.py
This time the Python script opens a Safari window and even if I execute it multiple times it opens the CodeFatherTech blog always in the same window (it doesn’t open a new tab).
I’m curious to see what happens if I:
- keep the code the same.
- change the URL I open in the script to ‘www.google.com’.
- keep the existing Safari tab open on the CodeFatherTech blog.
- run the updated script below.
import webbrowser
url = 'https://www.google.com'
webbrowser.open_new(url)
The Python script opens a second tab in Safari for Google and then it keeps opening Google in that second tab every time I run the script.
After using the open_new_tab() and open_new() functions I can see that the behaviour of these two functions is not always very clear.
As you can see below even the official documentation says that sometimes the function open_new_tab() is equivalent to open_new().

It can get confusing!?!
I’d rather stick to the generic open() function to make things simpler.
How Can I Open Chrome with Python?
So far we have seen how to open the default browser on your computer using Python.
At the moment on my computer the default browser is Safari but on your computer it could be a different one.
So, what if you want to tell Python to open a specific browser, for example Chrome?
You can use the webbrowser.get() function. This function returns a controller object that allows to select the type of browser to open.
Here is the object returned by this function on a Mac, try to execute this on your computer to see the type of object returned:
>>> import webbrowser
>>> webbrowser.get()
<webbrowser.MacOSXOSAScript object at 0x7fa5b0195970>
To find out the methods available on this object we can use the dir() built-in function.
>>> dir(webbrowser.get())
['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', '_name', 'args', 'open', 'open_new', 'open_new_tab']
Interesting…
You can see three methods that have very familiar names:
- open
- open_new
- open_new_tab
These are methods available on the controller object that have the same behaviour of the three functions with the same name available in the webbrowser module.
Let’s try to call the get() function followed by the open() method.
We are setting the value of the variable browser to ‘chrome’ considering that we want to open the URL in Chrome.
import webbrowser
url = 'https://codefather.tech/blog/'
browser = 'chrome'
webbrowser.get(browser).open(url)
When I execute the script it opens a new Chrome tab and it loads the CodeFatherTech website correctly.
To recap we have used the webbrowser get() function to change the browser we open the URL with.
Note: we have used a variable to define the browser we want to use so it’s easier to update the browser if we want to. On this page, you can find all the browsers available.
Let’s confirm that I can also open Safari if I change the value of the browser from ‘chrome’ to ‘safari’.
import webbrowser
url = 'https://codefather.tech/blog/'
browser = 'safari'
webbrowser.get(browser).open(url)
This worked fine on my machine.
Test it on yours too and if you don’t have a Mac select a browser from this list that exists on your machine.
Error When Opening a Web Browser in Python
I wonder what happens if I try to open a browser that is not installed on my computer.
Firefox (set browser variable to ‘firefox’)
$ python web_browser_test.py
70:78: execution error: Can’t get application "firefox". (-1728)
Opera (set browser variable to ‘opera’)
$ python web_browser_test.py
Traceback (most recent call last):
File "web_browser_test.py", line 5, in <module>
webbrowser.get(browser).open(url)
File "/Users/codefathertech/opt/anaconda3/lib/python3.8/webbrowser.py", line 65, in get
raise Error("could not locate runnable browser")
webbrowser.Error: could not locate runnable browser
We get back errors in both cases and the two errors are slightly different.
How Can You Open Multiple URLs in a Browser Using a Python Script?
So far we have seen how to open a single URL in a browser using Python.
And what if you want to create a Python script that opens multiple URLs?
You might want to do that if you want to create a script the does an initial setup of your environment.
For example, imagine you have a list of websites you want to open in multiple tabs because you need all of them as part of your job when you start working in the morning.
Let’s see how we can do it…
Use a Python list to store the list of websites and then use a for loop to open them one at a time.
import webbrowser
urls = ['codefather.tech/blog/', 'www.google.com', 'youtube.com', 'bbc.com']
browser = 'safari'
for url in urls:
webbrowser.get(browser).open(f"https://{url}")
Here is what we are doing in the script:
- import the webbrowser module.
- create a list of strings called urls that contains four websites.
- set the browser to Safari (change it to a browser that is installed on your machine and that currently is not open).
- use a for loop to go through the websites in the urls list.
- at every iteration of the four loop call webbrowser.get().open() to open one URL in the browser. To create the string we are passing to the open() method we are using f-strings to add https:// at the beginning of each URL.
Close the browser you are using for this test and execute the Python script.
And…
Magic!
The script opens a new browser window and loads the four websites in four different tabs.
Quite handy to save time, especially if you have a lot more websites you open regularly.
Running Python Command to Open a Browser
We have seen how to create a script that opens a web browser in Python.
And what if we want to open a web browser using just a single command without creating a Python script?
You can use the following syntax:
python -m webbrowser "https://codefather.tech/blog/"
We use the flag -m to specify the name of the Python module to use.
Conclusion
Python modules are so powerful!
Did you think you could have opened a web browser in Python with just a few lines of code?
And now that you know how to open one or more URLs in a browser using Python how will you use this knowledge?
This is just one of the modules that Python provides. To learn how modules work have a look at this tutorial about Python modules.
Also…
If you would like to become a better Python developer it’s important to understand how Python can be applied to different areas, for example, Data Science.
DataCamp has designed a course that teaches Data Science in Python, something that is becoming popular in today’s market.
Explore the DataCamp course Introduction to Data Science in Python today.
And Happy coding!
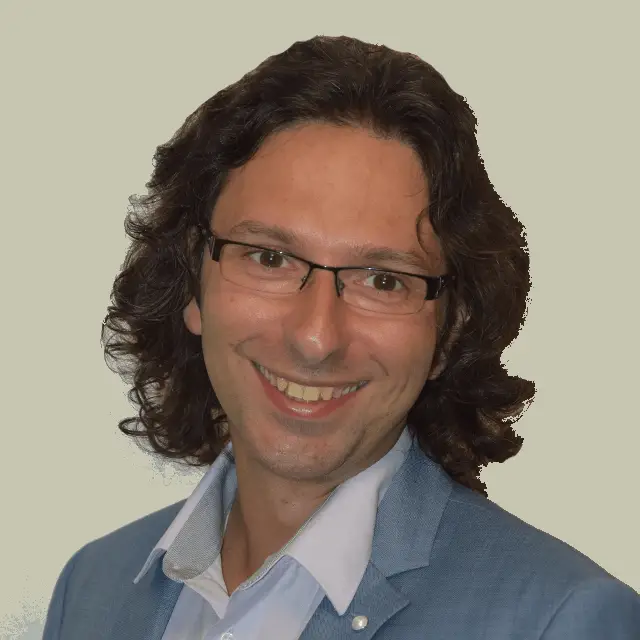
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.