Are you ready to dive into some Python magic? In this tutorial, we will explore how you can use lambda expressions to update every element in a Python list.
We will go through some cool examples to show you just how handy lambdas can be in handling list operations. So, open your IDE, and let’s get started!
How to Replace a For Loop with a Lambda and the Map Function
The basic approach you would use as a beginner developer to iterate through a list is with a for loop. But other options are available in Python.
In this section, we will see how lambdas can be very powerful when used to iterate through iterables like Python lists.
Let’s begin with a standard Python for loop that iterates through all the elements of a list of strings and creates a new list in which all the elements are uppercase.
countries = ['Italy', 'United Kingdom', 'Germany']
countries_uppercase = []
for country in countries:
countries_uppercase.append(country.upper())
print("Uppercase list: ", countries_uppercase)
Execute this code on your computer. You will see the following output:
Uppercase list: ['ITALY', 'UNITED KINGDOM', 'GERMANY']
Now we will write the same logic but using a lambda function and a Python built-in function called map() that has the following syntax:
map(function, iterable, ...)
The map function takes another function as the first argument and one or more iterables.
In this example, we will only pass one iterable (a list) to the map() function.
Have you ever seen a function that takes another function as an argument before?
A function that takes another function as an argument is called a Higher-Order Function.
It might sound complex, but this example will help you understand how it works.
So, what does the map() function do?
The map() function returns an iterable that is the result of the function passed as the first argument applied to every element of the iterable.
In our scenario, the function that we will pass as the first argument will be a lambda function that converts its argument into uppercase format.
Define a lambda function as shown below, you will pass this lambda as the first argument to the map() function:
lambda x: x.upper()
As iterable you will pass the countries list.
map(lambda x: x.upper(), countries)
Let’s execute this code in the Python shell:
>>> countries = ['Italy', 'United Kingdom', 'Germany']
>>> map(lambda x: x.upper(), countries)
<map object at 0x101477890>
You get back a map object, this is the standard type of object returned by the map() function. How can you get back a list instead?
To obtain a list when calling the map() function, you have to cast the map object to a list using the list() type.
>>> list(map(lambda x: x.upper(), countries))
['ITALY', 'UNITED KINGDOM', 'GERMANY']
You can see how using the map() function and a lambda function makes this code a lot more concise compared to the one using the for loop.
Another Example of Updating Every Element in a List with a Lambda Function
Imagine you have a list of strings representing names, and you want to format them into a more formal representation (e.g., “Mr. Tom Black”, or “Ms. Carol White”).
We will use a lambda function to add a title based on the gender of each person. The data structure you will work with is a list of tuples:
# List of names with gender
names = [
("Tom", "Black", "male"),
("Carol", "White", "female"),
("Alex", "Green", "male"),
("Emily", "Smith", "female")
]
# Lambda function to prepend title based on gender
format_name = lambda name: f"Mr. {name[0]} {name[1]}" if name[2] == "male" else f"Ms. {name[0]} {name[1]}"
# Use map() to apply the lambda function to each element of the list
formatted_names = list(map(format_name, names))
print(formatted_names)
Execute the Python code and you will see the following output:
['Mr. Tom Black', 'Ms. Carol White', 'Mr. Alex Green', 'Ms. Emily Smith']
In this example:
- We have a list
names
where each element is a tuple containing a name, surname, and gender. - The lambda function
format_name
checks the gender and prepends “Mr.” for males and “Ms.” for females. - We use
map()
to apply the lambda function to each element in thenames
list. - The result is a list of names formatted with the right titles.
Conclusion
In this tutorial, you have learned that Python offers multiple ways for iterating and modifying lists: the traditional for
loop and the more functional approach using map() combined with a lambda expression.
Lambdas, being anonymous and concise, pair perfectly with map() transforming lists with a single line of code.
Whether you choose the classic for
loop or the Pythonic map()
and lambda combination depends on your specific scenario and coding style. Knowing both methods gives you a deeper understanding of the Python programming language.
Related article: Would you like to have a stronger foundation about lambdas? If you haven’t checked it already, read the CodeFatherTech tutorial about Python lambda functions.
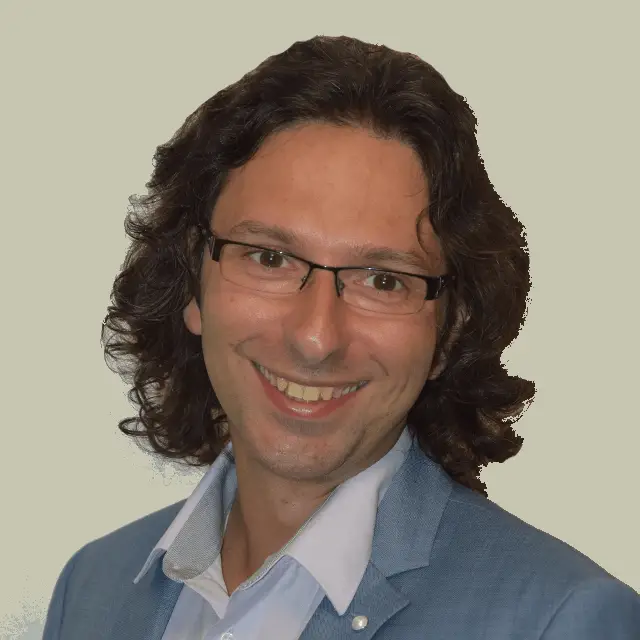
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.