A common requirement when you write a Python program is to be able to filter lists based on given conditions. This tutorial will show you how to filter a list with a lambda function in Python.
The applications of filtering lists are endless and we will go through examples that show you some of those.
How to Filter a List Using a Lambda Expression in Python
Python provides a built-in function that used with a lambda function allows you to filter a list based on specific conditions: the filter() function.
Below you can see the syntax of the filter() function that takes two arguments: the first argument is a function and the second argument is an iterable:
filter(function, iterable)
By using the filter() function, given a list, you can create a new list that contains only the elements in the original list that match a filtering condition. A lambda function allows you to define this condition.
For example, given a list of numbers, let’s see how to create a list that only includes the negative ones.
Here is the lambda function we will use:
lambda x : x < 0
Open the Python shell and execute this lambda passing a couple of numbers to it. This will show you how this lambda expression works.
>>> (lambda x : x < 0)(-1)
True
>>> (lambda x : x < 0)(3)
False
Note: in the code snippet above we have used parentheses to call our lambda function and pass an argument to it. If you want to know more about this syntax, go through the CodeFatherTech tutorial about the basics of lambdas in Python.
Our lambda function checks if a number is negative and returns a boolean with the following value:
- True if the argument is negative.
- False if the argument is positive.
Now, let’s apply the filter() function and this lambda to a list of integers to get only the negative ones:
>>> numbers = [1, 3, -1, -4, -5, -35, 67]
>>> negative_numbers = list(filter(lambda x : x < 0, numbers))
>>> negative_numbers
[-1, -4, -5, -35]
You get back the expected result, a list containing all the negative integers.
The filter function returns a list that contains a subset of the elements in the original list.
Examples of filter() and lambda Functions Used to Filter a List
Let’s have a look at a few more examples of filtering. These examples will give you more ideas on how to use Python lambdas for filtering lists.
Given a list of Linux commands return the ones starting with the letter ‘c’
>>> commands = ['ls', 'cat', 'find', 'echo', 'top', 'curl']
>>> list(filter(lambda cmd: cmd.startswith('c'), commands))
['cat', 'curl']
Generate a list of numbers with the Python range function and return the numbers greater than four:
>>> list(filter(lambda x: x > 4, range(15)))
[5, 6, 7, 8, 9, 10, 11, 12, 13, 14]
Filter Even Numbers from a List
>>> numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
>>> list(filter(lambda x: x % 2 == 0, numbers))
[2, 4, 6, 8, 10]
Filtering Words Longer Than a Certain Length
>>> words = ["lambda", "filter", "python", "list", "programming", "function"]
>>> list(filter(lambda w: len(w) > 6, words))
['programming', 'function']
FAQ
- Q: How does
filter()
differ from a list comprehension?- A: Both
filter()
and list comprehensions can create a new list based on conditions, but list comprehensions allow for more complex operations. They can also include aelse
clause, which is not supported byfilter()
.
- A: Both
- Q: Can I use the
filter()
function to modify the elements of a list, not just filter them?- A: No, the
filter()
function can only filter elements of a list based on a condition. If you want to modify elements, consider usingmap()
or a list comprehension.
- A: No, the
- Q: Is it possible to use
filter()
without passing a lambda function to it?- A: Yes, with
filter()
you can use any function that takes a single argument and returns a boolean value. This can be a built-in function or a user-defined function.
- A: Yes, with
- Q: How can I convert the result of the
filter()
function back to a list?- A: The
filter()
function returns an iterator. To convert it back to a list, you can use thelist()
constructor.
- A: The
- Q: What happens if the lambda function passed to the
filter()
function does not return a boolean value?- A: The
filter()
function expects the function it receives (including lambdas) to return a boolean value. Non-boolean return values are treated as truthy or falsey. In other words, non-empty strings, non-zero numbers, and other non-null objects are consideredTrue
. Empty strings, the number zero,None
are consideredFalse
.
- A: The
Conclusion
Well done for completing this tutorial!
Now you can start using the filter() and lambda functions to filter elements in a list based on what you need in your Python programs.
Make sure the examples in this tutorial on how to use filter() in Python are clear by trying them in your own Python shell or IDE.
Related article: As mentioned previously, if you want to have a deeper understanding of what lambda functions are, go through the introductory CodeFatherTech tutorial about lambda functions in Python.
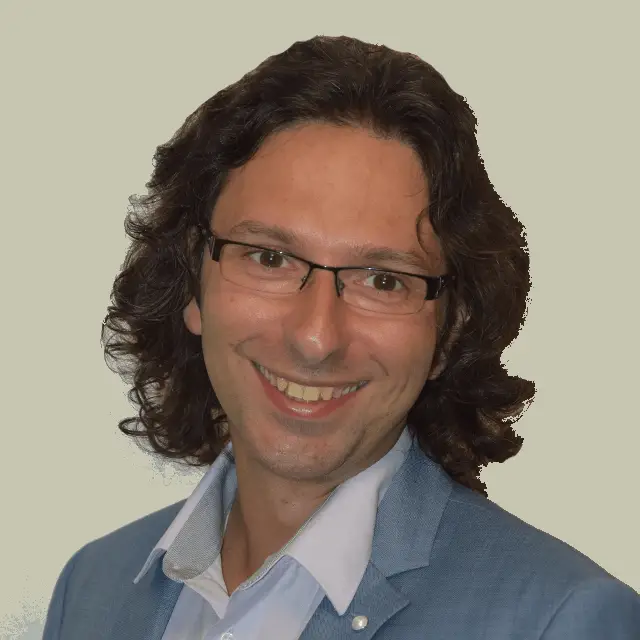
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.