Printing “Hello World” is usually the first thing a developer does when starting with a new programming language.
In this article, we will see how to print “Hello World” in Python.
The simplest way to print Hello World in Python is to pass a string to the print() function. The next step is to assign the string “Hello World” to a variable and then pass the variable to the print() function. This message can also be printed by using the + operator to concatenate two variables where the value of the first variable is “Hello” and the value of the second variable is “World”.
And these are just some ways to do that…
Let’s get creative and explore other possible ways!
1. How Do You Print Hello World in Python?
To print a message in Python you use the print() function. This is a function that receives a string as input and prints its value on the screen.
Let me explain…
Create a file called hello_world.py and add the following line of code to the file:
print("Hello World")
Note: you can create this file in a text editor or even better in an IDE like Visual Studio Code.
To run your program you can use the following command:
python hello_world.py
[output]
Hello World
We have specified the python command followed by the name of our Python program.
The extension .py identifies a file that contains Python code.
Important: in this tutorial, we are using Python 3.8. Also, in the following examples, we will always update the file hello_world.py with new code and we will execute our program using the Python command above.
2. Printing Hello World Using a Variable
In the previous section, we printed “Hello World” directly using the print() function.
This time I want to show you that it’s possible to assign the string “Hello World” to a variable first. Then after doing that you can print the value of the variable using the print() function.
Note: a variable allows storing data to be used in your program (in this case the string “Hello World”).
message = "Hello World using a variable"
print(message)
[output]
Hello World using a variable
Using the assignment operator ( = ) we have assigned the value on the right side of the operator to the variable message on its left side.
3. Concatenate two Python Strings to Print Hello World
We can also print our message by doing the following:
- Store the word “Hello” in a variable called word1
- Store the word “World” in a variable called word2
- Concatenate the two variables using the + operator
word1 = "Hello"
word2 = "World"
print(word1 + " " + word2)
Confirm that the output is “Hello World”.
Notice that we have concatenated one space character ” ” after word1 and before word2 to have a space between the words “Hello” and “World”.
Let’s see what happens if we remove that space character:
print(word1 + word2)
[output]
HelloWorld
We have removed the space between the two words.
4. Use the String format() Method to Print Hello World
Using the + operator to concatenate strings can get confusing when you try to create very long strings that contain several variables.
A cleaner option is to use the string format() method.
word1 = "Hello"
word2 = "World"
print("{} {}".format(word1, word2))
The first and second sets of curly brackets {} are replaced respectively by the values of the variables word1 and word2.
Let’s confirm the output is correct:
Hello World
It is correct!
5. Using Python f-strings to Print Hello World
With Python 3.6 and later you can use an alternative approach to the string format() method: Python f-strings.
Here is how it works…
word1 = "Hello"
word2 = "World"
print(f"{word1} {word2} using f-strings")
[output]
Hello World using f-strings
Notice the f letter just before the double quote.
This format is easier to read compared to the previous one considering that the variables word1 and word2 are embedded directly in the string.
Remember f-strings as they will definitely be useful in other Python programs.
6. Using a List and the String join() Method
So far we have used only strings…
In this section, we will introduce another data type: the list.
A list is used to store multiple values in a single variable. Lists are delimited by square brackets.
Firstly let’s assign the strings “Hello” and “World” to two items in our list called words.
words = ["Hello", "World"]
Then generate the message you want to print using the string join() method.
message = " ".join(words)
The join() method concatenates elements in a list (in this case our two words) using as a separator the string the join() method is applied to (in this case a single space ” “).
The result is that the worlds “Hello” and “World” are concatenated and an empty character is added between them.
print(message)
[output]
Hello World
Makes sense?
7. Using a List of Characters and the String join() Method
Let’s use a similar approach to the one in the previous section with the only difference being that in our list we don’t have words.
In our Python list, we have the individual characters of the string “Hello World”.
This example shows you that a Python string is made of individual characters.
characters = ['H','e','l','l','o',' ','W','o','r','l','d']
Notice that I have also included one space character between the characters of the two words.
Using the string join() method and the print() function we will print our message.
message = "".join(characters)
print(message)
[output]
Hello World
Nice!
8. Using Dictionary Values to Print Hello World in Python
But wait, there’s more!
In this section and in the next one we will use a different Python data type: the dictionary.
Every item in a dictionary has a key and a value associated with that key. A dictionary is delimited by curly brackets.
Let’s create a dictionary called words…
words = {1: "Hello", 2: "World"}
Here is how you can read this dictionary:
- First item: key = 1 and value = “Hello”
- Second item: key = 2 and value = “World”
We can generate the string “Hello World” by passing the values of the dictionary to the string join() method.
To understand how this works let’s print first the value of words.values().
print(words.values())
[output]
dict_values(['Hello', 'World'])
The values of the dictionary are returned in a list that we can then pass to the string join() method.
message = " ".join(words.values())
print(message)
[output]
Hello World
Test it on your computer to make sure you understand this syntax.
9. Using Python Dictionary Keys to Print Hello World
Once again in this section, we will use a dictionary…
The difference compared to the previous example is that we will store the strings “Hello” and “World” in the keys of the dictionary instead of using the values.
Our dictionary becomes…
words = {"Hello": 1, "World": 2}
Let’s go through this dictionary:
- First item: key = “Hello” and value = 1
- Second item: key = “World” and value = 2
This time let’s see what we get back when we print the keys of the dictionary using words.keys().
print(words.keys())
[output]
dict_keys(['Hello', 'World'])
Once again we get back a list.
At this point, we can concatenate the strings in the list of keys using the string join() method.
message = " ".join(words.keys())
print(message)
Verify that your Python program prints the correct message.
How Do You Print Hello World 10 Times in Python?
One last thing before completing this tutorial…
In your program, you might need to print a specific string multiple times.
Let’s see how we can do that with the message “Hello World”.
print("Hello World"*10)
Very simple…
I have used the * operator followed by the number of repetitions next to the string I want to repeat.
And here is the output:
Hello WorldHello WorldHello WorldHello WorldHello WorldHello WorldHello WorldHello WorldHello WorldHello World
Conclusion
Well done for completing this tutorial!
And if this is one of your first Python tutorials congratulations!!
To recap, we have covered a few Python basics here:
- Variables
- Data types (strings, lists, and dictionaries)
- print() function
- + operator to concatenate strings
- string methods .format() and .join()
- f-strings
It can be quite a lot if you are just getting started with Python so you might have to go through this tutorial a few times to make all these concepts yours.
Bonus read: we have used lists a few times in this tutorial. Go through another tutorial that will show you how to work with Python lists.
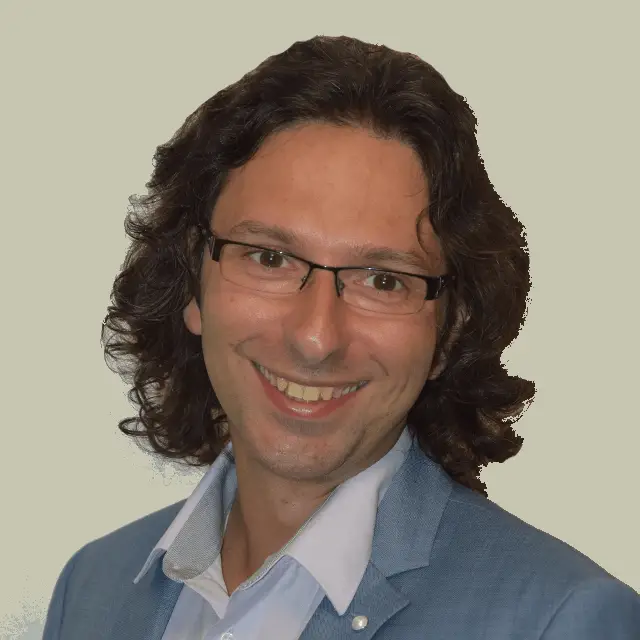
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.