Printing even and odd numbers from a Python list is a good exercise if you are getting started with Python and you want to learn its basics.
To extract even and odd numbers from a Python list you can use a for loop and the Python modulo operator. A second option is to replace the for loop with a list comprehension. The extended syntax of the slice operator also allows to do that with one line of code but only when you have lists of consecutive numbers.
We will go through few examples in this tutorial and the point of implementing multiple ways to solve the same problem is to grow the creativity you need as Python developer.
Let’s get started!
How Do You Print Odd Numbers From a List in Python?
To print odd numbers from a list of numbers you can use the Python modulo operator, related to the mathematical concept of remainder.
When you divide an odd number by 2 the remainder of the division is 1. When you divide an even number by 2 the remainder of the division is 0.
Let’s use this concept and a Python for loop to print odd numbers from a list.
def get_odd_numbers(numbers):
odd_numbers = []
for number in numbers:
if number % 2 == 1:
odd_numbers.append(number)
return odd_numbers
Before starting the for loop we define an empty list, then at every iteration of the for loop we add odd numbers to the list.
numbers = [2, 3, 6, 8, 13, 45, 67, 88, 99, 100]
print(get_odd_numbers(numbers))
[output]
[3, 13, 45, 67, 99]
How Do You Print Even Numbers From a List in Python?
This is very similar to what we have seen in the previous section…
To print even numbers from a list of numbers we can extract the numbers that divided by 2 return a remainder equal to 0.
The code is identical to the one we have created to extract odd numbers with a small difference in the if condition inside the for loop.
def get_even_numbers(numbers):
even_numbers = []
for number in numbers:
if number % 2 == 0:
even_numbers.append(number)
return even_numbers
And the output of the function is…
numbers = [2, 3, 6, 8, 13, 45, 67, 88, 99, 100]
print(get_even_numbers(numbers))
[output]
[2, 6, 8, 88, 100]
How to Create a Function That Splits Odd and Even Numbers in a List
To split odd and even numbers in a list we could use the same approach based on the for loop used previously and generate two different lists, one for odd and one for even numbers.
Instead of doing that I want to make our code more concise by using list comprehensions.
We will still use the concept of remainder…
Let’s create a basic function that takes a list as argument and returns two lists, one that contains odd numbers and one that contains even numbers.
def split_list_even_odd(numbers):
odd_numbers = [number for number in numbers if number % 2 == 1]
even_numbers = [number for number in numbers if number % 2 == 0]
return odd_numbers, even_numbers
Notice that this function returns two Python lists.
Do you know in what format are these lists received by the caller of the function?
Let’s find out…
numbers = [2, 3, 6, 8, 13, 45, 67, 88, 99, 100]
print(split_list_even_odd(numbers))
[output]
([3, 13, 45, 67, 99], [2, 6, 8, 88, 100])
The function caller receives a tuple with two elements. We can access each of them by using square brackets and an index.
print("The odd numbers are {}".format(split_list_even_odd(numbers)[0]))
print("The even numbers are {}".format(split_list_even_odd(numbers)[1]))
[output]
The odd numbers are [3, 13, 45, 67, 99]
The even numbers are [2, 6, 8, 88, 100]
If you want to know more about the .format() syntax used in the print statement you can have a look at this tutorial about concatenating Python strings.
Program To Print Even and Odd Numbers From 1 to 100
To print even and odd numbers from 1 to 100 we could first create our list of numbers manually but it would take ages!
Instead we will use the Python range() function.
Here is how you can generate numbers from 1 to 100 using the Python range() function.
>>> numbers = range(1,101)
>>> print(type(numbers))
<class 'range'>
Interestingly the type of the object returned by the range() function is not a list.
So, what can we do with it?
We can still use a for loop or a list comprehension to go through all the numbers…
>>> [number for number in numbers]
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99, 100]
Use the function we have created before to print even and odd numbers.
def split_list_even_odd(numbers):
odd_numbers = [number for number in numbers if number % 2 == 1]
even_numbers = [number for number in numbers if number % 2 == 0]
return odd_numbers, even_numbers
numbers = range(1,101)
print("The odd numbers are {}".format(split_list_even_odd(numbers)[0]))
print("The even numbers are {}".format(split_list_even_odd(numbers)[1]))
[output]
The odd numbers are [1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21, 23, 25, 27, 29, 31, 33, 35, 37, 39, 41, 43, 45, 47, 49, 51, 53, 55, 57, 59, 61, 63, 65, 67, 69, 71, 73, 75, 77, 79, 81, 83, 85, 87, 89, 91, 93, 95, 97, 99]
The even numbers are [2, 4, 6, 8, 10, 12, 14, 16, 18, 20, 22, 24, 26, 28, 30, 32, 34, 36, 38, 40, 42, 44, 46, 48, 50, 52, 54, 56, 58, 60, 62, 64, 66, 68, 70, 72, 74, 76, 78, 80, 82, 84, 86, 88, 90, 92, 94, 96, 98, 100]
How Do You Print Odd Numbers From a List of Consecutive Numbers in Python?
The simplest way to print odd numbers from a list of consecutive numbers is with the extended syntax of the slice operator.
The slice operator returns a fragment of a list given a start index (included) and an end index (not included).
list[start_index:end_index]
Let’s create a list that contains the numbers from 1 to 10:
>>> numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
To get the first 4 elements of the list with the standard slice operator syntax we can use the following expression.
>>> numbers[0:4]
[1, 2, 3, 4]
Note: as mentioned before the end index is not included in the list returned by the slice operator.
To get back a full list using the slice operator you can use the following syntax:
>>> numbers[:]
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Ok, but how does this help us retrieve only odd numbers?
For that we have to add something to the basic syntax of the slice operator, that’s why it’s called extended syntax.
With the extended syntax you can pass a third argument to the slice operator, this arguments defines the step between the items to be retrieved from the list.
list[start_index:end_index:step]
For example, if the value of the step is 2 the slice operator will retrieve every 2 numbers.
Now that we know this we are ready to retrieve odd numbers from our list…
>>> numbers[::2]
[1, 3, 5, 7, 9]
We basically get the full list back because we haven’t provided start and end index. Additionally we only get odd numbers because we have specified a step equal to 2.
How Do You Print Even Numbers From a List of Consecutive Numbers in Python?
This is very similar to what we have seen in the previous section…
To print even numbers from a Python list of consecutive numbers you can use the extended slice syntax with start index equal to 1, empty end index and step equal to 2.
>>> numbers[1::2]
[2, 4, 6, 8, 10]
Et voilà!
This is pretty simple once you get used to the syntax of the slice operator.
Python Program to Print the Largest Even and Largest Odd Number in a List
To print the largest even and odd number in a Python list we can start from the concepts we have seen before related to the slice operator.
The additional thing we have to do is to apply the max() function to the list returned by the slice operator.
Largest Odd Number
>>> max(numbers[::2])
9
Largest Even Number
>>> max(numbers[1::2])
10
Nice and simple 🙂
Develop a Python Program that Counts Even and Odd Numbers in a List Provided by a User
Before completing this tutorial let’s see how we can use the Python input function to get the list of numbers from the user input.
First of all I want to see what kind of data type we receive from the user input.
>>> numbers = input("Please provide a list of numbers (separated by space): ")
Please provide a list of numbers (separated by space): 1 3 6 37 56 23 89 103 346
>>> print(numbers)
1 3 6 37 56 23 89 103 346
>>> print(type(numbers))
<class 'str'>
The user input is a single string so we have to do a bit of string manipulation to convert it into a list first.
The Python string split() method allows to convert a string into a list.
>>> print(numbers.split())
['1', '3', '6', '37', '56', '23', '89', '103', '346']
That’s a step forward, but we still have a list of strings and we want a list of integers instead.
A list comprehension and the Python int() function can be used to convert a list of strings into a list of integers:
>>> numbers = ['1', '3', '6', '37', '56', '23', '89', '103', '346']
>>> [int(number) for number in numbers]
[1, 3, 6, 37, 56, 23, 89, 103, 346]
Nice 🙂
And now we are ready to create our function that converts the string provided by the user into a list of integers.
def get_integers(numbers):
return [int(number) for number in numbers.split()]
After converting the user input into a list of integers we can call the split_list_even_odd() function created before.
Here is the full program:
def get_integers(numbers):
return [int(number) for number in numbers.split()]
def split_list_even_odd(numbers):
odd_numbers = [number for number in numbers if number % 2 == 1]
even_numbers = [number for number in numbers if number % 2 == 0]
return odd_numbers, even_numbers
numbers = input("Please provide a list of numbers (separated by space): ")
numbers = get_integers(numbers)
print("The odd numbers are {}".format(split_list_even_odd(numbers)[0]))
print("The even numbers are {}".format(split_list_even_odd(numbers)[1]))
Let’s execute our program…
Please provide a list of numbers (separated by space): 1 3 6 34 56 43 67 88 101 102 134
The odd numbers are [1, 3, 43, 67, 101]
The even numbers are [6, 34, 56, 88, 102, 134]
It works!!
Conclusion
If you had any doubts about extracting even and odd numbers from a Python list before reading this article…
…now you have all the knowledge you need to do it.
If you feel a few things are still unclear go back and rewrite the examples in this tutorial from scratch by yourself.
Practice is the best way to learn and remember Python constructs.
Happy coding!! 😀
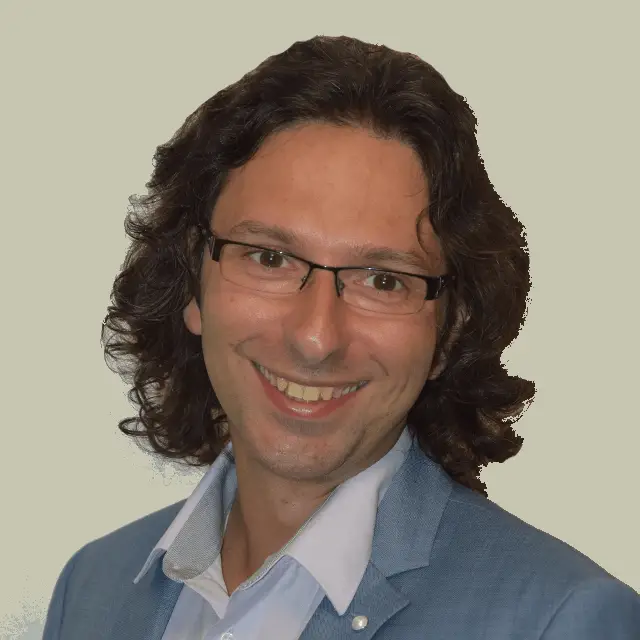
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.