The square root of a number x is the number y whose square is equal to x. In mathematics, the square root is represented by “√”.
In this tutorial, we will see how to calculate the square root of a number in Python using multiple approaches.
You can use several Python modules to calculate the square root of a number and you can also define your own function to calculate the square root.
Let’s get started.
How Do you Find the Square Root in Python?
The math module gives access to a wide range of mathematical functions. You don’t need to install this module separately because it is already part of the Python installation. Use the sqrt() function of the math module which returns the square root of a number.
import math
square_root = math.sqrt(49)
print(square_root)
Output:
7.0
After importing the math module, we call the sqrt() function and pass a number to it. This function returns the square root of the number passed to the function.
Note that we are passing an integer value, we can also pass a float value.
import math
square_root = math.sqrt(10.5)
print(square_root)
Output:
3.24037034920393
The value returned by the math.sqrt() function is still a float.
How Can You Calculate the Square Root of a Python List?
Python has some very powerful data types and one of them is the list. Lists are used to store multiple values in a single variable, they are ordered and mutable. You can define lists using square brackets.
Let’s see what happens if we use the Python math module to calculate the square root of a list…
import math
numbers = [10, 20, 30.50, 40.30, 50.80]
square_root = math.sqrt(numbers)
print(square_root)
When you execute this code you get the following exception that says that the math sqrt() function doesn’t support lists as input.
Traceback (most recent call last):
File "square_root.py", line 8, in <module>
square_root = math.sqrt(numbers)
TypeError: must be real number, not list
There is an alternative way to use math.sqrt() to calculate the square root of a list, we can use it inside a Python for loop.
import math
numbers = [10, 20, 30.50, 40.30, 50.80]
square_root = []
for number in numbers:
square_root.append(math.sqrt(number))
print(square_root)
First, we define an empty list that will contain the square root of each value in the original list. Then in every iteration of the for loop, we calculate the square root of one value at a time and we append the result to the square_root list.
The output is…
[3.1622776601683795, 4.47213595499958, 5.522680508593631, 6.348228099241551, 7.127411872482185]
Let’s make this code shorter…
You can calculate the square root of the values in a list using the sqrt() function part of the NumPy module. NumPy can manipulate arrays and numbers, and it has to be installed separately from your Python installation.
To install NumPy use the following command in your command prompt/shell:
pip install numpy
After installing NumPy, you can use it to calculate the square root of a list.
import numpy as np
numbers = [10, 20, 30.50, 40.30, 50.80]
square_root = np.sqrt(numbers)
print(square_root)
This time, we are importing NumPy and passing a list to the sqrt() function. This function returns the square roots of all the values in the original list.
Note that we are passing integer and float values in the same list, this is because lists support multiple data types.
[3.16227766 4.47213595 5.52268051 6.3482281 7.12741187]
Can You Calculate the Square Root of Arrays Using NumPy?
We have seen how to calculate the square root of lists.
Let’s see how to calculate the square root of NumPy arrays…
The code below initializes a NumPy array and calculates its square root.
import numpy as np
numbers = np.array([10,20,30,40,50])
square_root = np.sqrt(numbers)
print(square_root)
Output:
[3.16227766 4.47213595 5.47722558 6.32455532 7.07106781]
We have initialized an array and then passed it to the NumPy sqrt() function.
Calculate the Square Root in Python Without the Math Module
You can also calculate the square root using the Python built-in function pow(). This function takes two parameters, the first one is the base and the second one is the exponent.
pow(base, exp)
To calculate the square root of the base, use 0.5 as the exponent.
Let’s write a function that takes a value as input and returns the square root of this value using the pow() function.
import math
def sqrt(value):
return math.pow(value, 0.5)
print(sqrt(49))
Execute this code on your computer and verify that the output is 7.0.
Python Square Root Using the Exponent Operator
And here is another way to calculate the square root of a number…
The exponent operator (**) can be used to calculate the square root of a number in Python.
Similarly to what we have done in the previous section define a function that calculates the square root. This time we will use the exponent operator instead of the pow() built-in function.
def sqrt(value):
return value ** 0.5
print(sqrt(49))
We have defined a function that takes a value as input and returns the square root of the number using the exponent operator.
Execute this code and verify that the output is 7.0.
Calculate the Square Root of a Complex Number Using the CMath module
The cmath module allows manipulating complex numbers in Python. This module is built-in so there is no need to install it separately.
Define a function that returns the square root of a complex number.
import cmath
def sqrt(value):
return cmath.sqrt(value)
print(sqrt(49 + 30j))
Output:
(7.295694952809256+2.0560070146880456j)
The function above takes a complex number as input and returns its square root using the sqrt() function of the cmath module.
Conclusion
Using Python, it is very easy to calculate the square root of a number. The math module is easy to use and NumPy also allows calculating the square root of lists and arrays.
The cmath module is good to work with complex numbers.
You now know multiple ways to calculate the square root of a number in Python as part of your programs.
Bonus read: In this article, we have defined a few functions, these are the building blocks of your programs. Learn more about creating your functions using Python.
Related course: Build your Python foundations with this beginner Python course from the University of Michigan.
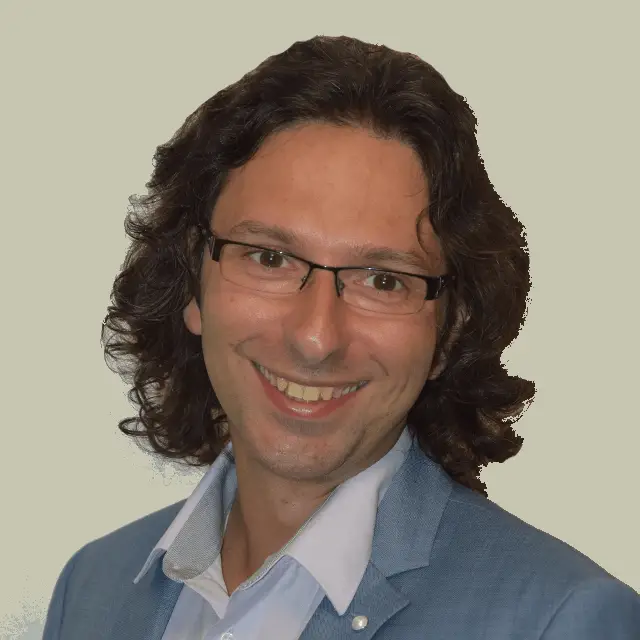
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.