In this tutorial we will calculate the absolute value of numeric types in Python. We will also look at different libraries that allow to calculate it.
To calculate the absolute value of a number in Python you can use the abs() built-in function. The argument passed to the abs function can be an integer, a float or a complex number. Python modules like NumPy and Pandas also allow to calculate the absolute value for more complex data structures.
It’s time for some examples!
The Absolute Value Function in Python
The simplest way to get the absolute value of a number in Python is with the built-in function abs().
Let’s write some Python code that takes a number as input and returns the absolute value of that number:
number = int(input("Please insert a number: "))
print("The absolute value of the number is {}".format(abs(number)))
In this case we have made the assumption that our program is expecting integers. The output is:
Please insert a number: -4
The absolute value of the number is 4
Now, open the Python shell to see the absolute value returned by the abs() function when we pass different types on numbers to it.
Integer number
>>> abs(4)
4
>>> abs(-4)
4
Floating point number
>>> abs(4.15)
4.15
>>> abs(-4.15)
4.15
You can see that with int and float numbers we get back either an int or a float without sign.
Absolute Value of a Complex Number
Here is what happens with a complex number…
>>> abs(3+2j)
3.605551275463989
>>> abs(3-2j)
3.605551275463989
>>> abs(3-4j)
5.0
>>> abs(3+4j)
5.0
The absolute value returned for a complex number is its magnitude. The magnitude is the distance of the complex number from the origin in the complex plane.
Notice how the magnitude of a complex number can have a decimal part different than zero or not.
In one of the next sections we will see a math Python library that you can also use to calculate the absolute value.
Absolute Value of the Elements in a Python List
Let’s move to something more interesting…
I want to find a way to calculate the absolute value of all the elements that belong to a Python list.
How can we do it?
There are few ways, we can start with a basic for loop:
>>> numbers = [1, -3, -3.14, 6+3j, -11, 5]
>>> abs_numbers = []
>>> for number in numbers:
... abs_numbers.append(abs(number))
...
>>> abs_numbers
[1, 3, 3.14, 6.708203932499369, 11, 5]
I have created a new list and appended the absolute value of each number to it.
Easy…and there are better ways to do it.
Map Function
Run the following in the Python shell to check the manual for the map function:
>>> help(map)
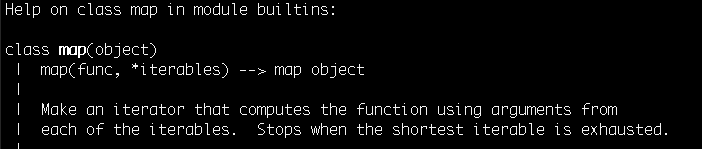
In this case the iterable will be a list of numbers:
>>> numbers = [1, -3, -3.14, 6+3j, -11, 5]
>>> map(abs, numbers)
<map object at 0x10d9d5050>
>>> list(map(abs, numbers))
[1, 3, 3.14, 6.708203932499369, 11, 5]
When we execute the map statement we get back a map object. To get a list back we have to cast it to a list using the list() function.
List Comprehension
And here is how we can use a list comprehension to get a list that contains absolute values of all these numbers.
>>> numbers = [1, -3, -3.14, 6+3j, -11, 5]
>>> [abs(number) for number in numbers]
[1, 3, 3.14, 6.708203932499369, 11, 5]
Nice!
Lambda
I also want to try using a lambda for this. The lambda will change the sign of a number if it’s negative:
>>> list(map(lambda x: -x if x < 0 else x, numbers))
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "<stdin>", line 1, in <lambda>
TypeError: '<' not supported between instances of 'complex' and 'int'
Unfortunately, this doesn’t work with our current list which contains a complex number.
Let’s test this approach with a list that doesn’t contain any complex numbers:
>>> numbers2 = [1, -3, -3.14, -11, 5]
>>> list(map(lambda x: -x if x < 0 else x, numbers2))
[1, 3, 3.14, 11, 5]
Looks good this time 🙂
Absolute Value of the Elements in a Tuple or a Set
I’m pretty sure I can calculate the absolute value of the elements in a Python tuple or set using one of the techniques used in the previous section.
Let’s confirm it for a tuple first…
>>> numbers = (1, -3, -3.14, 6+3j, -11, 5)
>>> tuple(map(abs, numbers))
(1, 3, 3.14, 6.708203932499369, 11, 5)
And here’s the same for a Python set:
>>> numbers = {1, -3, -3.14, 6+3j, -11, 5}
>>> set(map(abs, numbers))
{1, 3.14, 3, 5, 6.708203932499369, 11}
Notice the difference in the order of the elements of the set we have calculated. This is due to the fact that a set is an unordered collection.
Calculate the Absolute Value with NumPy
An alternative to the abs() built-in function to calculate the absolute value of a number is the NumPy module.
>>> import numpy as np
>>> np.abs(-2)
2
>>> np.abs(-3.14)
3.14
>>> np.abs(6+3j)
6.708203932499369
So far this works like the built-in method so what’s the point of using this instead?
We can also pass a list directly to the NumPy abs() function…
>>> numbers = [1, -3, -3.14, 6+3j, -11, 5]
>>> np.abs(numbers)
array([ 1. , 3. , 3.14 , 6.70820393, 11. ,
5. ])
>>> np.absolute(numbers)
array([ 1. , 3. , 3.14 , 6.70820393, 11. ,
5. ])
Note: the absolute function in NumPy is the same as abs. It was firstly introduced in NumPy and then the alias abs was created for simplicity.
Now, I’m curious to see what happens when we pass a list to the built-in abs() function:
>>> abs(numbers)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: bad operand type for abs(): 'list'
Ok, it definitely doesn’t work!
Absolute Value of a Matrix in Python
Considering that we had a look at NumPy in the previous section, let’s see if we can also calculate the absolute value of the elements of a matrix.
>>> x = np.matrix('1 -2 3-2j; -4.34 5 -6.54')
>>> np.abs(x)
matrix([[1. , 2. , 3.60555128],
[4.34 , 5. , 6.54 ]])
It works with a matrix too. Quite handy!
Absolute Value for a Pandas Dataframe
And now that we have used NumPy…
…we can also try the same with a Pandas dataframe?
>>> df = pd.DataFrame({"Int": [1, -3, -6], "Float": [-3.14, -4.56, 5.55], "Complex": [3-2j, -4+3j, -3-9j]})
>>> df
Int Float Complex
0 1 -3.14 3.000000-2.000000j
1 -3 -4.56 -4.000000+3.000000j
2 -6 5.55 -3.000000-9.000000j
>>> np.abs(df)
Int Float Complex
0 1.0 3.14 3.605551
1 3.0 4.56 5.000000
2 6.0 5.55 9.486833
The result is a dataframe in which every element is exactly the absolute value we were expecting.
Who would have thought we could have done so many things with the absolute value? 🙂
Sorting the Values of a Dictionary Based on the Absolute Value
This might not necessarily be something you will encounter in the future, but it’s an exercise to improve your Python skills.
How can we sort the elements of a dictionary based on the absolute value of their values?
>>> my_dict = {'number1': -3, 'number2': -5.45, 'number3': 2, 'number4': -10}
The first thing we need is the sorted function.

If we simply apply the sorted function to the dictionary we get the keys ordered alphabetically:
>>> sorted(my_dict)
['number1', 'number2', 'number3', 'number4']
This is not what we want…
We want those keys ordered based on the absolute value of the values mapped to them in the dictionary.
As you can see from the help above the sorted function allows to pass a key that can be used as sorting criteria.
Firstly, we will define a lambda function that takes a dictionary key as input and returns the absolute value of the dictionary value associated with that key.
>>> (lambda key : abs(my_dict[key]))('number1')
3
In the code above I have defined the lambda function and then I have passed the string ‘number1’ to it. In this way I have tested the logic of the lambda.
Now we have to use this lambda as a sorting key for the sorted function.
>>> my_dict = {'number1': -3, 'number2': -5.45, 'number3': 2, 'number4': -10}
>>> sorted(my_dict, key=lambda key : abs(my_dict[key]))
['number3', 'number1', 'number2', 'number4']
If we want to reverse the order we can set the argument reverse of the sorted function to True.
>>> sorted(my_dict, key=lambda key : abs(my_dict[key]), reverse=True)
['number4', 'number2', 'number1', 'number3']
Time Difference Between Dates using the Absolute Value
In your Python program, you might want to calculate the difference in seconds between two dates.
Let’s say we are only interested in the absolute value of the difference between the two.
>>> import datetime, time
>>> date1 = datetime.datetime.now()
>>> time.sleep(10)
>>> date2 = datetime.datetime.now()
>>> delta = abs(date1 - date2)
>>> delta
datetime.timedelta(seconds=25, microseconds=287887)
>>> delta = abs(date2 - date1)
>>> delta
datetime.timedelta(seconds=25, microseconds=287887)
Using the datetime module we get the current date in two different moments (notice the 10 seconds sleep that adds some extra delay).
We get the same result when we calculate the difference between the two delta values because we have applied the absolute value to both differences.
Here is what we get without applying the absolute value:
>>> delta = date1 - date2
>>> delta
datetime.timedelta(days=-1, seconds=86374, microseconds=712113)
>>> delta = date2 - date1
>>> delta
datetime.timedelta(seconds=25, microseconds=287887)
Conclusion
In this tutorial we have covered a few ways of calculating the absolute value in Python using the Python standard library and modules like NumPy and Pandas.
We have also seen the flexibility that Python provides in solving the same problem in multiple ways.
I hope you have found what you were looking for and let me know in the comments if you would like to see something else in this article I haven’t already covered.
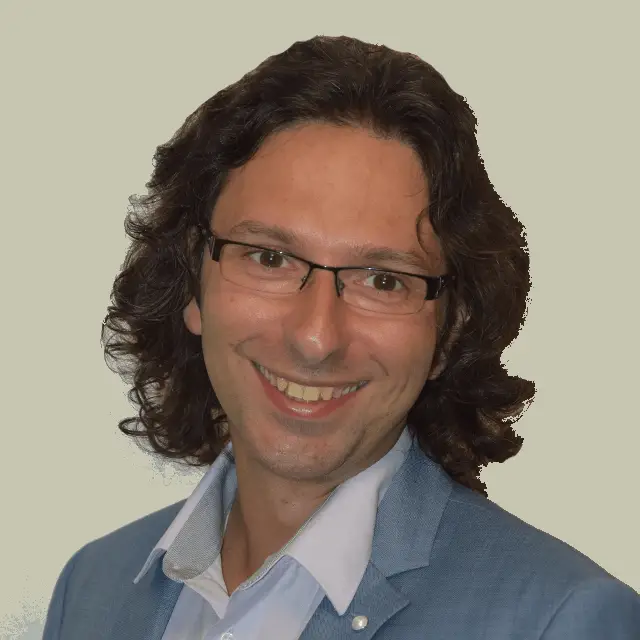
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.