In this article you will learn how to create a while loop in Bash.
While loops are used in Bash scripting and in many other programming languages…
Let’s create a loop that goes through N numbers and prints only the odd ones.
You will see how our script looks like if we hardcode the value of N in it, and then you will learn how to pass the value of N to the script as an argument via the Linux command line.
This applies to any Bash shell running on Unix-like systems (including MacOS).
First of all let’s have a look at the generic structure of a while loop in Bash:
while [ condition ]
do
command1
command2
...
commandN
done
Here is how a while loop works…
As long as the condition between square brackets is true the commands between do and done are executed.
How does this apply to this specific case?
Print Odd Numbers Using a Bash Script
INDEX=0
while [ INDEX < N ]
do
if [ $INDEX is Odd ]
then
print $INDEX
fi
increase $INDEX
done
And if we translate this logic into an actual Bash script…
#!/bin/bash
INDEX=0
while [ $INDEX -lt 20 ]
do
REMAINDER=$(( $INDEX % 2 ))
if [ $REMAINDER -ne 0 ]
then
echo $INDEX
fi
INDEX=$(($INDEX+1))
done
Let’s review what this script does:
- Set the value of the variable INDEX to 0.
- Start a while loop that is executed if the value of INDEX is less than 20.
- At every iteration of the loop calculate the REMAINDER for the division of the INDEX by 2.
- Check if the REMAINDER is not zero…in that case print INDEX because it’s an odd number
- Increment the value of INDEX by 1 before executing the next iteration of the while loop
Note: Arithmetic operations can be executed in a Bash script using $(( )).
Here is what we see if we execute it:
myuser@localhost:~$ ./print_odd_numbers.sh
1
3
5
7
9
11
13
15
17
19
It works well!
The Bash script does print the odd numbers from 1 to 20.
Passing N as an Argument Through the Command Line
We now want to pass N via the command line.
To do that we will have to apply the following changes:
- Use a variable called N and assign to it the first argument passed to the script
- Replace 20 with N in the condition of the while loop
#!/bin/bash
N=$1
INDEX=0
while [ $INDEX -lt $N ]
do
REMAINDER=$(( $INDEX % 2 ))
if [ $REMAINDER -ne 0 ]
then
echo $INDEX
fi
INDEX=$(($INDEX+1))
done
What is $1?!?
It’s the variable that in Bash contains the first argument passed to a script.
Execute the script again, this time passing N via the command line:
myuser@localhost:~$ ./print_odd_numbers.sh 20
1
3
5
7
9
11
13
15
17
19
The script works well, so our changes are correct 🙂
There are other ways to implement a loop in Bash, see how you can write a for loop in Bash.
Conclusion
In this tutorial you have learned:
- The structure of a while loop in Bash.
- How to use an if statement nested in a while loop.
- The way you can use the arithmetic operator to calculate the remainder of a division.
- How an index variable can be used in a while loop.
- The technique to pass Bash script arguments via the command line.
Also, sometimes you might have the need to delay some of the commands executed in your while loops. To do that you would use the Bash sleep command.
And now, what else will you create with this knowledge? 😀
Related FREE Course: Decipher Bash Scripting
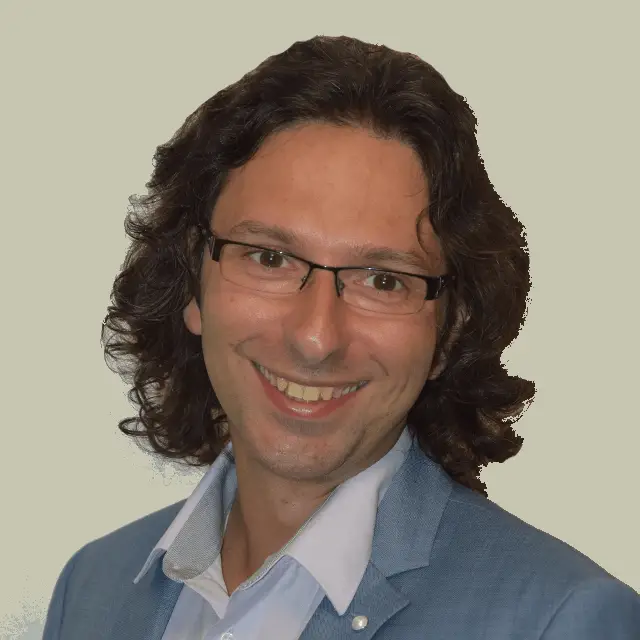
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.
I need to generate even no >100 starting 10 to n
INDEX=10
while [ $INDEX < 200 ]
do
REMAINDER=$(( $INDEX % 2 ))
if [ $REMAINDER -eq 0 ]
then
echo $INDEX
fi
INDEX=$(($INDEX+2))
done
Thank you Ruchir.
while [ $INDEX < 200 ] should be while [ $INDEX -lt 200 ]