Variables in Python allow you to store and manipulate data to be used in your programs.
In Python, a variable is like a name tag you give to a value. When you create a variable, you are telling Python to remember a value for you so you can use it later.
Think of it as giving a label to a specific piece of information. This makes your code easier to read and work with.
A variable doesn’t store the actual value itself. Instead, it points to the value. For example:
x = 5
Here, x
refers to an integer object with the value 5
. Whenever you use x
, Python knows you mean 5
. This concept works for all types of data in Python, like numbers, strings, and lists.
How Assignments Work in Python
The single line of code shown in the previous section is an example of assignment.
In Python, an assignment is the process of binding a value to a variable. It is done using the assignment operator (=
). Once a variable is assigned a value, that variable can be used to refer to the value in your code.
Here you can see the generic syntax for an assignment in Python:
variable_name = value
Previously we have seen how to create an integer variable. Now let’s see how to create a string and a list.
# Example of string
word = "hello"
# Example of list
words = ["hello", "goodbye"]
Working with Multiple Assignments
You can assign values to multiple variables with one line of code:
a, b, c = 1, 2, 3
This is equivalent to the following code:
a = 1
b = 2
c = 3
You can also use Python’s assignment operator to swap values between variables:
a, b = b, a
This line of code swaps the values between the variables a
and b
. Let’s verify it using the Python shell:
>>> a = 10
>>> b = 45
>>> a, b = b, a
>>> a
45
>>> b
10
You can see that the values have been swapped correctly.
Using “Good” Variable Names
You should be using meaningful names for Python variables. This will make you code clear, readable, and easier to maintain for yourself and other developers in the future.
A variable name should reflect its purpose and the data it holds, making the code easier to understand without requiring too many comments. For instance, using product_price
instead of pp
or x
is self-explanatory for a developer reading or updating the code.
In my career as a developer I have seen many examples of “bad” variable names that make the code harder to read. Imagine you have two variables:
- The first variable holds a success message.
- The second variable holds a failure message.
Below you can see a poor choice for variable names:
message1 = "Your request is successful" # Success message
message2 = "Your request has failed" # Failure message
The poor variable choice forces you to add comments to the code.
A good choice for the name of these variables is the following:
success_message = "Your request is successful"
failure_message = "Your request has failed"
Another way to improve readability is to use the same case for all the variables in your program. An example is snake_case (that we have used in the code above), where each space is replaced by an underscore character.
Here are examples of variables using snake case for 5 different data types:
# Integer
total_price = 20
# Float
max_temperature = 33.5
# String
first_name = "Jane"
# List
healthy_foods = ["apple", "tomato", "banana"]
# Dictionary
student_ages = {"Peter": 21, "Jack": 24, "Kate": 20}
Now you know the basics to start using Python variables and assigning values to them.
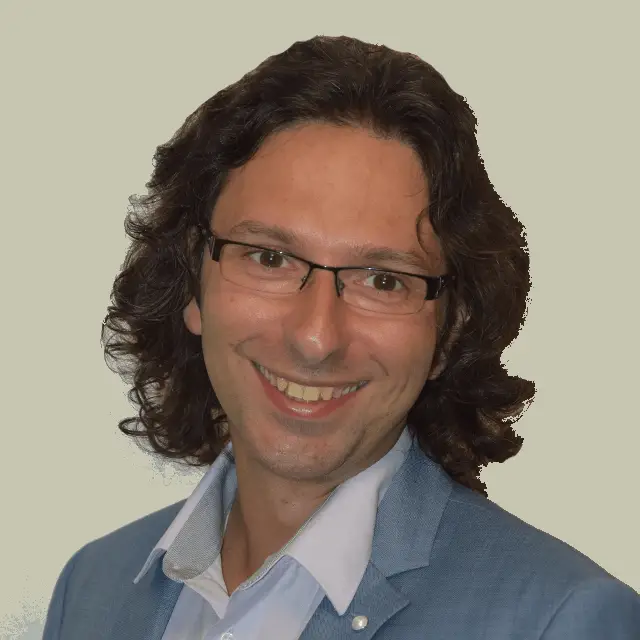
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for helping others become Software Engineers.