Imagine you are creating an application in which you have to track multiple users and each user has several properties (e.g. name, surname, and age). You could use a list of dictionaries as a data type to handle this requirement.
You can represent the properties related to one user using the following dictionary:
user1 = {'name': 'John', 'surname': 'Red', 'age': 27}
To track the properties of multiple users you can define a list of dictionaries:
users = [
{'name': 'John', 'surname': 'Red', 'age': 27},
{'name': 'Kate', 'surname': 'Green', 'age': 40},
{'name': 'Jack', 'surname': 'Brown', 'age': 32}
]
Notice that every item in the list is a dictionary. Items in this list are separated by a comma.
Let’s print the variable users
and confirm its type using the type()
built-in function:
print(users)
[output]
[{'name': 'John', 'surname': 'Red', 'age': 27}, {'name': 'Kate', 'surname': 'Green', 'age': 40}, {'name': 'Jack', 'surname': 'Brown', 'age': 32}]
print(type(users))
[output]
<class 'list'>
When printing the list of dictionaries, the output shows open and closed square brackets and within those, all the dictionaries are separated by commas.
To print a list of dictionaries without brackets you can use a Python for loop.
for user in users:
print(user)
[output]
{'name': 'John', 'surname': 'Red', 'age': 27}
{'name': 'Kate', 'surname': 'Green', 'age': 40}
{'name': 'Jack', 'surname': 'Brown', 'age': 32}
{'name': 'Frank', 'surname': 'Black', 'age': 36}
This makes the output a lot more readable as you can see the dictionaries one under the other.
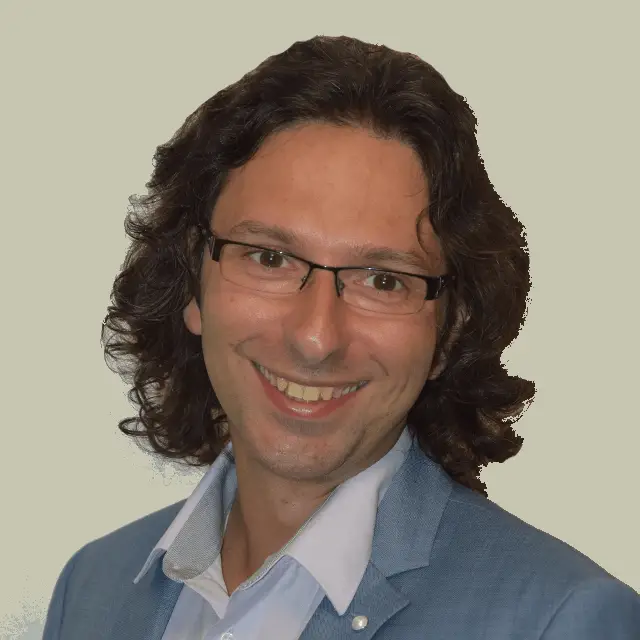
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for helping others become Software Engineers.