Knowing how to compare strings in Python is a must if you want to be a Python programmer.
In this guide you will learn:
- How to verify if two strings are equal or different
- A way to see which string between two comes first alphabetically
- If string comparisons are case sensitive
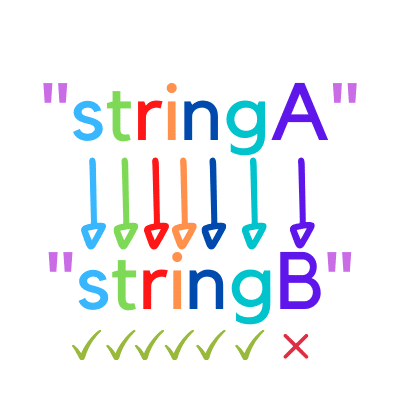
First of all, Python compares strings character by character.
Characters in the same position are read from both strings and compared.
This is done behind the scenes by Python using the Unicode value of each character, you don’t really see that…
And if you don’t know what the Unicode value is don’t worry about it right now.
One important thing to know is that Python comparison operators return True or False.
The following table shows the string comparison operators explained in this guide:
Operator | What does it do? |
string1 == string2 | Checks if two strings are equal |
string1 != string2 | Checks if two strings are not equal |
string1 < string2 | Checks if string1 comes before string2 alphabetically |
string1 > string2 | Checks if string1 comes after string2 alphabetically |
If you come back to this table after reading the full guide it will help you remember each comparison operator and how to use it.
Let’s see some practical examples!
Basic String Comparison in Python
A basic scenario in which you would compare two strings is when you want to understand if two strings are identical or which one between two strings comes first alphabetically.
For instance, I’m writing a program and I declare a variable called programming_level that represents the level of programming knowledge of a user that is using our Python program.
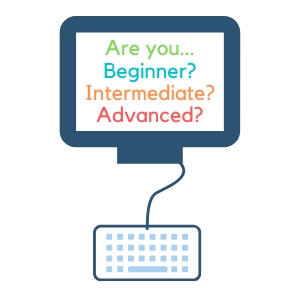
At the beginning of the program, I ask the user to specify the level between three choices: beginner, intermediate and advanced.
To get an input from the user I use the input() function that sets the value of the programming_level variable based on the value provided by the user.
I also want to make sure the user cannot provide other values outside of those three, I do that using a Python if else statement and the Python logical and operator.
I create a Python program called strings.py:
programming_level = input("Please specify you programming level (beginner / intermediate / advanced): ")
if programming_level != "beginner" and programming_level != "intermediate" and programming_level != "advanced":
print("The programming level specified is invalid: %s" % programming_level)
else:
print("Thank you! Welcome to our %s programming training" % programming_level)
As you can see, to verify that the value specified by the user is correct I make sure it’s not different from all the three choices: beginner, intermediate and advanced.
How do I do that?
Using the Python comparison operator != that checks if two strings are not equal.
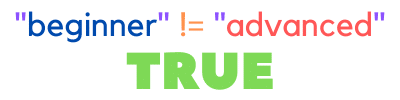
If two strings are not equal the comparison operator != returns True, otherwise it returns False.
And if I run the program passing beginner as level:
$ python strings.py
Please specify you programming level (beginner / intermediate / advanced): beginner
Thank you! Welcome to our beginner programming training
I get back the “Thank you” message.
Let’s try to pass an incorrect value…
Please specify you programming level (beginner / intermediate / advanced): alien
The programming level specified is invalid: alien
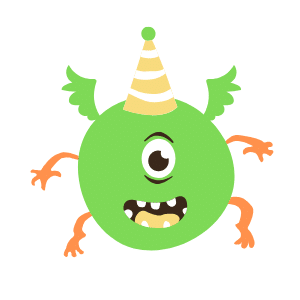
In this case, the program says that the programming level specific is invalid.
Wondering if aliens know Python? 🙂
In Python we can also check if two strings are equal, let’s see how….
Python String Equality Check
The Python equality operator ( == ) is used to check if two strings are equal.
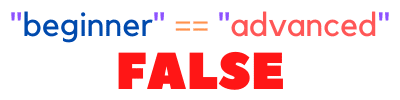
If the two strings are equal the equality operator returns True, otherwise it returns False.
I will modify the previous program to use the equality operator, to do that I need to swap the logic of the if else statement.
programming_level = input("Please specify you programming level (beginner / intermediate / advanced): ")
if programming_level == "beginner" or programming_level == "intermediate" or programming_level == "advanced":
print("Thank you! Welcome to our %s programming training" % programming_level)
else:
print("The programming level specified is invalid: %s" % programming_level)
Can you see what has changed?
- The if logic is now using the == operator and the Python or logical operator to check if the value of the programming_level variable is either beginner, intermediate or advanced.
- I have swapped the two print statements considering that I will print the “Thank you” message if the if condition is true.
This second version makes more sense to me logically compared to the first version.
What do you think?
Try to run the program, its output won’t change.
Do you have any questions so far?
Compare Strings Alphabetically in Python
Python provides other operators that allow to check which one between two strings comes first alphabetically (< and >).
For example, I want to see which one between “beginner” and “intermediate” comes first alphabetically.
I create a program called alphabetical.py:
programming_level_1 = "beginner"
programming_level_2 = "intermediate"
if programming_level_1 < programming_level_2:
print("%s comes before %s" % (programming_level_1, programming_level_2))
else:
print("%s comes after %s" % (programming_level_1, programming_level_2))
The program returns:
$ python alphabetical.py
beginner comes before intermediate
So, now you know how to compare two strings to get their alphabetical order.
Is Python String Comparison Case Sensitive?
Now, I wonder if string comparison in Python is case-sensitive or not.
What do you think?
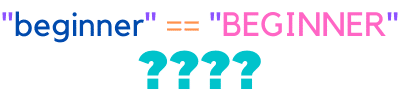
We will print the boolean value of an expression using the equality operator that compares the string “beginner” with the string “BEGINNER”:
programming_level_1 = "beginner"
programming_level_2 = "BEGINNER"
print(programming_level_1 == programming_level_2)
Let’s run it:
$ python case_sensitive.py
False
So, the two strings are not equal, hence…
Python string comparison is case-sensitive.
But, wait…
What if you want to do a case-insensitive string comparison…how can you do it?
You can use the lower() function applied to each string.
Our small program becomes:
programming_level_1 = "beginner"
programming_level_2 = "BEGINNER"
print(programming_level_1.lower() == programming_level_2.lower())
And the output is:
$ python alphabetical.py
True
So this time the two strings are considered identical because the string comparison, with the lower() function applied to both strings, is case insensitive.
Finally, let’s look at which one comes first between the strings “Beginner” and “beginner”.
Any guess?
We can confirm it with two simple lines of Python (in theory just one of them would be enough):
print("beginner" < "Beginner")
print("beginner" > "Beginner")
That returns…
False
True
So “beginner” (with lower case b) is considered bigger than “Beginner” (with upper case b).
Good to know to avoid bugs in our programs!
Conclusion
Now you know enough to compare strings in your Python programs.
There’s so much you can do just with strings…
…for example, you could read a document programmatically and verify if there are any grammatical errors by simply comparing each word in the document with the words in a dictionary file.
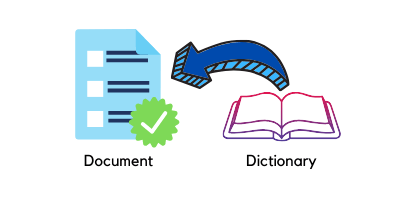
And that’s just the first thing that comes to my mind right now.
The ways you can apply this knowledge are endless!
And if you are just getting started with Python, I created a newsletter you can’t miss for you to quickly learn the basics of Python. You can join the Python Codefather newsletter for free here.
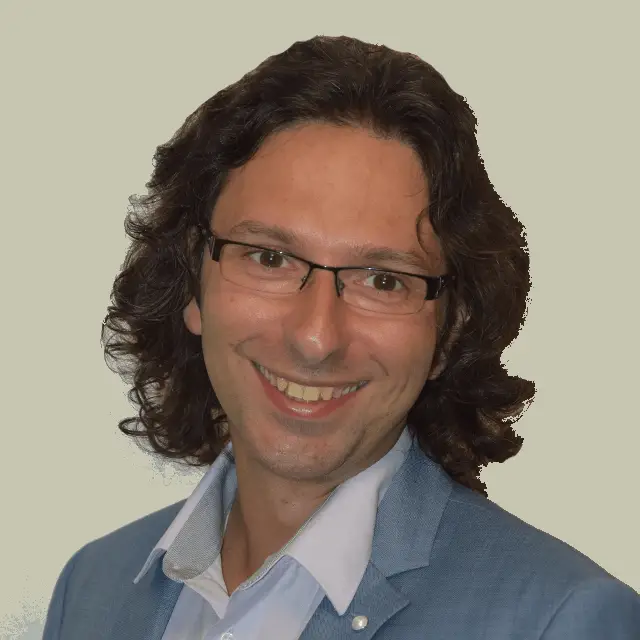
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.