Knowing how to write the elements of a list to a file in Python can be handy. This tutorial will show you how to do that in multiple ways.
A common approach to writing list elements to a file using Python is to first iterate through the elements of the list using a for loop. Then use a file object to write every element of the list to the file as part of each loop iteration. The file object needs to be opened in write mode.
There are many scenarios in which writing the items of a list to a file can turn out useful.
Let’s have a look at a few examples!
Python Program to Write a List to a File in Python Using a Loop
Imagine you are building an application that has to persist a list of items after its execution.
This might be required, for example, if you store data in a file every time you run your application and that data can be available for later use the next time you run your application.
Let’s have a look at how you can take the list of strings below and store each string in a text file using Python.
months = ['January', 'February', 'March', 'April']
In the first Python program, we will open a file object in write mode and then use a for loop to write each element in our data structure to the file:
output_file = open('months.txt', 'w')
for month in months:
output_file.write(month)
output_file.close()
Using the Python open() built-in function we open a new file in write mode. Each iteration of the for loop writes an element of the list months to the file. We write to the text file using the write method of the file object.
Then, close the file object once you have written all the elements of the list to it.
Now, let’s have a look at what the content of the file looks like using the cat command (or any text editor).
$ cat months.txt
JanuaryFebruaryMarchApril
It looks like we are missing newline characters at the end of each line, let’s add a newline to the write method in each iteration of the for loop:
output_file = open('months.txt', 'w')
for month in months:
output_file.write(month + '\n')
output_file.close()
The output looks a lot better now:
$ cat months.txt
January
February
March
April
What Error Do You See When Writing a List into a File Opened in Read Mode?
I want to see what happens if we don’t open the file in write mode.
Replace the following line in your Python code:
output_file = open('months.txt', 'w')
With the following…
output_file = open('months.txt')
Notice that we have removed the second argument passed to the open() function that was telling the function to open the file in write mode.
Here is the error we get when we run our code with Python 3 if we don’t delete the months.txt file created in the previous example:
$ python write_list_to_file.py
Traceback (most recent call last):
File "write_list_to_file.py", line 6, in <module>
output_file.write(month + '\n')
IOError: File not open for writing
The error tells us that the file has not been opened for writing. Without passing the second parameter to the open() function we have opened the file in read mode.
If you are using Python 2 you will see the following error:
IOError: File not open for writing
If you delete the file months.txt and rerun the program you get a different error.
Python 2
Traceback (most recent call last):
File "write_list_to_file.py", line 3, in
output_file = open('months.txt')
IOError: [Errno 2] No such file or directory: 'months.txt'
Python 3
Traceback (most recent call last):
File "write_list_to_file.py", line 3, in <module>
output_file = open('months.txt')
FileNotFoundError: [Errno 2] No such file or directory: 'months.txt'
The Python interpreter tries to open the file in read mode but considering that the file doesn’t exist we get back the “No such file or directory” error.
Method to Write a List to a File Using the With Open Statement
Instead of remembering to close the file object after the end of the for loop, we can use the Python with open statement.
The “with open” statement creates a context manager and this syntax automatically closes the file object when you don’t need it anymore.
Here is how our Python code becomes…
months = ['January', 'February', 'March', 'April']
with open('months.txt', 'w') as output_file:
for month in months:
output_file.write(month + '\n')
A lot more concise!
Execute it and confirm that every element in the list is written to the file as expected.
Syntax to Write a List to File Using the String Join Method
I wonder if I can replace the for loop with a single line that uses the string join() method.
The string join method joins the elements of a sequence into a single string where every element is separated from another by the string the join method is applied to.
Maybe it’s easier to show it in the Python shell 🙂
>>> months = ['January', 'February', 'March', 'April']
>>> '#'.join(months)
'January#February#March#April'
I have used the # character in this example because in this way it’s easier to see how the # is used to separate elements in the list.
Notice that the separation character # doesn’t appear after the last element.
So, this is the result if we use this approach in our program and replace the # character with the newline character:
output_file = open('months.txt', 'w')
output_file.write('\n'.join(months))
output_file.close()
The result is:
$ cat months.txt
January
February
March
April$
The dollar at the end of the last line is the shell itself considering that the new line character is not present at the end of the last line.
So, this method kind of works but it’s not as good as the for loop because it doesn’t add a new line character in the last line of the file.
Write List to File in Python Using Writelines
Python’s file object provides a method called writelines() that writes a list of lines to a stream, for example, a file object.
It feels like it could be another good way to do this, let’s give it a try!
output_file = open('months.txt', 'w')
output_file.writelines(months)
output_file.close()
The writelines method takes a list and writes the list content to a file using a single statement.
Here is the output when I run the program:
cat months.txt
JanuaryFebruaryMarchApril$
Hmmm…this doesn’t look right.
The file writelines() method doesn’t add any line separators.
I wonder why?!?
What we could do is add the newline character after each element in the list and then pass the list to the writelines method.
Let’s use a list comprehension to do that:
output_file = open('months.txt', 'w')
months = ["{}\n".format(month) for month in months]
output_file.writelines(months)
output_file.close()
You can see that we are also using the string format() method to add the new line character at the end of each string in the list.
The output we get back looks good:
$ cat months.txt
January
February
March
April
How to Write a Python List to a File Using a Lambda
The final approach to write the strings in our list to a file will use a lambda function and the map function.
Using lambdas is optional, we are doing it in this tutorial as an exercise to refresh more advanced Python concepts.
Below you can see the syntax of the map function:
map(function, iterable, ...)
The map function applies the function passed as the first parameter to every item of the iterable.
In this case, the function will be a lambda function that appends the newline character to a given string and writes the result to a file object.
We will use this as a generic approach to write every item in the list followed by a newline to the output file.
Here is the lambda function to use:
lambda x: output_file.write(x + '\n')
And now let’s pass the lambda to the map function:
months = ['January', 'February', 'March', 'April']
output_file = open('months.txt', 'w')
map(lambda x: output_file.write(x + '\n'), months)
output_file.close()
Let’s confirm if the output is correct…
$ cat months.txt
January
February
March
April
Yes, it is!
How to Write a List to a File Using the Python Pickle Module
So far, we have seen how to write a list to a text file with the idea that the text file should be human-readable.
Now, we will assume that the file we write to only has to be read by a Python application and not by a person.
If that’s the case, you can use the Python pickle module to write the list content to the file (serialize a list).
import pickle
months = ['January', 'February', 'March', 'April']
months_file = open('months.out', 'wb')
pickle.dump(months, months_file)
months_file.close()
After opening the file object we use the function pickle.dump to write the list to the file (serialize the list object).
The value of the mode argument we pass to the built-in function open() is ‘wb‘. This means that we are opening the file for writing in binary mode.
Here is what the content of the binary file written to disk looks like:
��*]�(�January�February��March��April�e.%
You can then read the list back into memory from the file using the function pickle.load after opening the file for reading in binary mode (rb).
months_file = open('months.out', 'rb')
months = pickle.load(months_file)
months_file.close()
print(months)
[output]
['January', 'February', 'March', 'April']
I have created a separate tutorial if you want to learn more about Python pickle.
How do You Write a List to a JSON file in Python?
You might also want to write the entire list to a JSON file. To do that you can use the Python json module.
We will use the function json.dumps() to write the list to the file. We will call the function json.loads() to read the list back from the JSON file.
import json
months = ['January', 'February', 'March', 'April']
json_file = open('months.json', 'w')
json_file.write(json.dumps(months))
json_file.close()
Here is the content of the file you created:
["January", "February", "March", "April"]
To read the list object back from the JSON file you can use json.loads(). This function takes as an argument the JSON data read from the file.
json_file = open('months.json', 'r')
months = json.loads(json_file.read())
print(months)
I have created a more in-depth tutorial if you want to learn more about the Python json module.
Conclusion
We have seen so many different approaches to writing a Python list to a text file. Including how to write and read a file using pickle Python’s module.
It has been an excellent exercise to practice multiple ways of saving data to a file using Python.
Related articles: read the following CodeFatherTech articles to build more in-depth knowledge about:
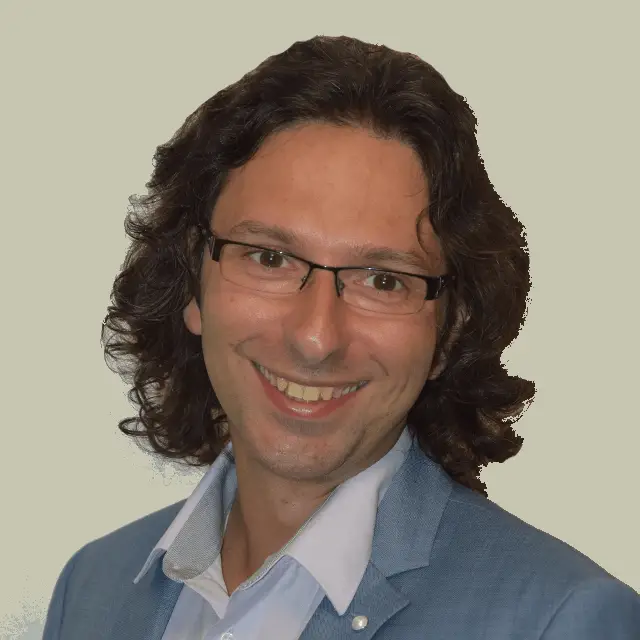
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.