I want to calculate the remainder of the division between two numbers in Python, how can I do it?
The Python Modulo operator returns the remainder of the division between two numbers and is represented using the % symbol. The Modulo operator belongs to Python’s arithmetic operators. Here is an example of how to use it: 5 % 2 is equal to 1 (the remainder of the division between 5 and 2).
The symbol of the modulo operator is % and you have to specify it between the two operands of the division.
Here are some examples of how to use it:
>>> 5 % 2
1
>>> 8 % 3
2
>>> 8 % 2
0
Let’s explain the examples above:
- 5 modulo 2 is 1 because 5 divided by 2 is 2 with a remainder of 1.
- 8 modulo 3 is 2 because 8 divided by 3 is 2 with a remainder of 2.
- 8 modulo 2 is 0 because 8 divided by 2 is 4 with a remainder of 0.
The modulo operator is sometimes called modulus or Python modulo division.
How can I calculate the Python modulo of negative integers?
Previously we have seen how the modulo works for positive integers.
Let’s test it on negative integers:
>>> -5 % 2
1
>>> -5 % -2
-1
>>> 5 % -2
-1
You can see that the Python modulo returns a remainder of the same sign as the divisor.
Calculating the Python modulo of a float
Now, we will see how the modulo works with floating-point numbers.
>>> 3.4 % 2
1.4
>>> 3.4 % 2.0
1.4
>>> 3.4 % 2.2
1.1999999999999997
The first two examples work the same way we have seen with integers.
Let’s confirm it’s the case also for the third example:
>>> 3.4 / 2.2
1.5454545454545452
>>> 3.4 - 2.2
1.1999999999999997
So, 3.4 % 2.2 is 1.1999999999999997 because 3.4 equals 1*2.2 + 1.1999999999999997.
>>> 1*2.2 + 1.1999999999999997
3.4
Calculating the modulo if the dividend is smaller than the divisor
In the previous examples, the dividend (left side of the modulo operator) was always bigger than the divisor (right side of the modulo operator).
Now, we will see what happens if the dividend is smaller than the divisor.
>>> 2 % 10
2
>>> 3 % 5
3
In both examples, the result of the division is 0, that’s why the modulo is equal to the value of the dividend.
What about with floats?
>>> 2.2 % 10.2
2.2
>>> 3.4 % 6.7
3.4
That’s the same for floats.
Can I use the Python modulo inside an if statement?
One typical use of the Python modulo operator is a program that given a list of numbers prints odd or even numbers.
This is based on the following logic:
- An odd number divided by 2 gives a remainder of 1.
- An even number divided by 2 gives a remainder of 0.
>>> for x in range(20):
… if x % 2 == 0:
… print("The number {} is even".format(x))
… else:
… print("The number {} is odd".format(x))
…
The number 0 is even
The number 1 is odd
The number 2 is even
The number 3 is odd
The number 4 is even
The number 5 is odd
The number 6 is even
The number 7 is odd
The number 8 is even
The number 9 is odd
The number 10 is even
The number 11 is odd
The number 12 is even
The number 13 is odd
The number 14 is even
The number 15 is odd
The number 16 is even
The number 17 is odd
The number 18 is even
The number 19 is odd
Notice that to print the value of x as part of the print statement we have used the string format() method.
Python modulo when the divisor is zero
Here is what happens when the divisor of an expression using the modulo operator is zero.
For integers:
>>> 3 % 0
Traceback (most recent call last):
File "", line 1, in
ZeroDivisionError: integer division or modulo by zero
For floats:
>>> 2.4 % 0
Traceback (most recent call last):
File "", line 1, in
ZeroDivisionError: float modulo
In both cases, the Python interpreter raises a ZeroDivisionError exception.
You can handle the exception using a try-except statement.
>>> try:
… 3 % 0
… except ZeroDivisionError:
… print("You cannot divide a number by zero")
…
You cannot divide a number by zero
Using the Math.fmod Function
An alternative to the % modulo operator which is preferred when working with floats, is the fmod() function part of the Python math module.
Below you can see the description of math.fmod() from the Python official documentation.
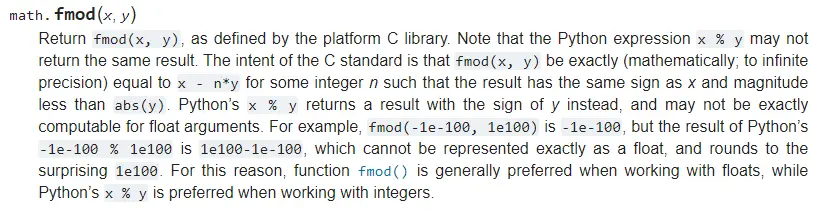
Before we have tested the % operator with negative integers:
>>> -5 % 2
1
>>> -5 % -2
-1
>>> 5 % -2
-1
Let’s see the results we get using math.fmod():
>>> math.fmod(-5, 2)
-1.0
>>> math.fmod(-5, -2)
-1.0
>>> math.fmod(5, -2)
1.0
And here is the difference when we calculate the modulo with negative floats.
Modulo Operator used with negative floats
>>> -5.2 % 2.2
1.4000000000000004
>>> -5.2 % -2.2
-0.7999999999999998
>>> 5.2 % -2.2
-1.4000000000000004
Math fmod Python Function used with negative floats
>>> math.fmod(-5.2, 2.2)
-0.7999999999999998
>>> math.fmod(-5.2, -2.2)
-0.7999999999999998
>>> math.fmod(5.2, -2.2)
0.7999999999999998
Now that we have gone through several examples that show how to use the modulo operator, let me know in the comments what are you using the modulo for.
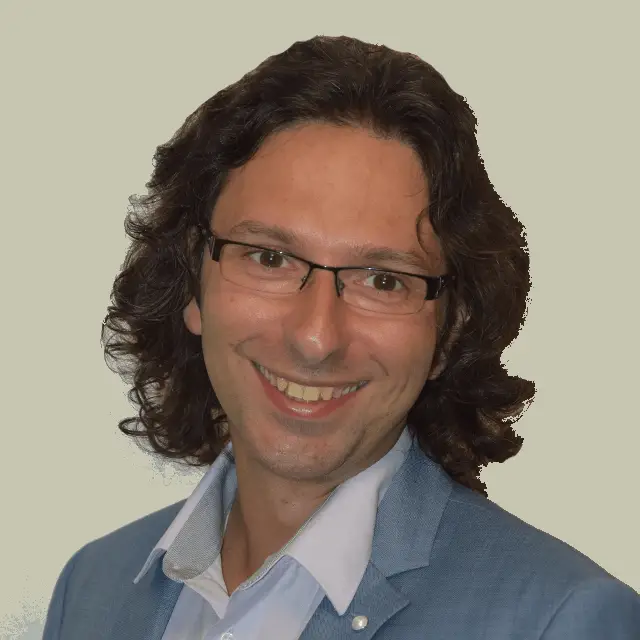
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.