When you first start learning Python, there’s one feature that immediately stands out from other programming languages: indentation.
Indentation is not just a style preference, it’s a core part of Python’s syntax. Misunderstanding how it works can lead to a frustrating debugging experience, but once you understand its logic, it becomes a clean and intuitive way to write your code.
Why Does Python Rely on Indentation?
Unlike many languages that use braces {}
or keywords (e.g., BEGIN
and END
) to define blocks of code, Python uses indentation to determine the structure of your code. This was an intentional design choice by Python’s creator, Guido van Rossum, to promote readability. Python’s motto, “Readability counts” is not just a cliché. Properly indented code resembles an outline, making your code easier to scan and debug.
But the reliance on indentation isn’t only about aesthetics. A consistent indentation prevents ambiguous block definitions. However, mastering this system takes more than simply pressing the Tab key, it involves understanding its rules.
Python enforces indentation for all blocks of code, such as function definitions, loops, and conditionals. Let’s examine an example of correctly and incorrectly indented code:
# Correctly indented code
def say_hello(name):
if name:
print(f"Hello, {name}!")
else:
print("Hello!")
# Incorrectly indented code
def say_hello(name):
if name:
print(f"Hello, {name}!")
else:
print("Hello!")
In the incorrect example, the if
statement is not indented. Here is what happens when I try to execute the code that is not indented correctly (write the code in a file called indentation.py
).
# python3 indentation.py
File "/opt/codefather/indentation.py", line 3
if name:
^
IndentationError: expected an indented block after function definition on line 2
Python will raise an IndentationError
as soon as it encounters the missing indentation. Unlike other languages where your code might still compile with minor formatting errors, Python says, “Nope!”.
This approach ensures everyone reading your code sees the same clear structure.
A Common Pitfall: Tabs vs. Spaces
If you’re just starting out, you might not give much thought to whether you’re using tabs or spaces for indentation. But in Python, mixing the two can lead to errors difficult to troubleshoot.
I will write the following code using:
- 8 spaces on the line that prints the message “This line uses spaces”.
- 1 tab on the line that prints the message “This line uses tabs”.
Try it out on your computer as well:
# Code mixing tabs and spaces
def example():
if True:
print("This line uses spaces")
print("This line uses tabs")
While this code might look OK in your editor, Python will throw an IndentationError
.
# python3 mixing_tabs_and_spaces.py
File "/Users/claudiosabato/Documents/Coding/Python/tutorials/mixing_tabs_and_spaces.py", line 5
print("This line uses tabs")
TabError: inconsistent use of tabs and spaces in indentation
Python has a strong preference for spaces over tabs, and the official Python Style Guide (PEP 8) recommends using 4 spaces for each level of indentation. Many modern IDEs (like PyCharm or VSCode) offer settings to help enforce this standard, so take advantage of those tools.
Indentation in Nested Blocks
When transitioning to more advanced Python concepts, you’ll encounter scenarios requiring multiple nested blocks. Here’s an example using nested loops:
# Nested indentation done right
for i in range(5):
for j in range(3):
print(f"i: {i}, j: {j}")
Each new block requires an extra level of indentation. But nested blocks are where many beginners can get confused.
Ensure each nested section is clearly aligned. Avoid deeply nested structures where possible, as they reduce readability. Consider refactoring with functions to flatten the logic.
# Refactoring deep nesting with functions
def process_j(i):
for j in range(3):
print(f"i: {i}, j: {j}")
for i in range(5):
process_j(i)
Indentation Checking Tools
Even with careful attention, mistakes happen. That’s where tools can help you. Many IDEs and text editors, such as VSCode and PyCharm, include live linters that underline problematic code as you type.
Static code analysis tools like Flake8 or Pylint can catch indentation errors before you run your script.
If you’re working on a shared project, a code formatter like Black can automatically apply consistent indentation and formatting to your files.
Indentation as a Best Practice, Not Just a Requirement
It’s easy to think of Python’s indentation rules as a hurdle to clear on your way to writing functional code. But once you internalize its benefits, indentation evolves into one of Python’s strengths.
While enforcing blocks is necessary, it also encourages you to write clean, structured, and highly readable code from day one. This self-discipline carries over into other languages and makes you a better developer overall.
While teaching programming languages to beginners, one of the things I have noticed is a tendency to ignore indentation. The result is code that is messy and hard to read or maintain.
With Python the bad habit of ignoring indentation gets addressed immediately because Python doesn't allow you to execute code with incorrect indentation.
Key Takeaways
- Python’s reliance on indentation enforces readable, well-structured code.
- Avoid mixing tabs and spaces. Stick to 4 spaces for each level of indentation as per PEP 8.
- Leverage IDE settings to catch indentation errors early.
- Refactor deeply nested blocks with functions to improve readability and maintainability of your code.
Mastering Python indentation is not just about avoiding syntax errors, it’s about adopting a mindset that enables you to write clean and maintainable code.
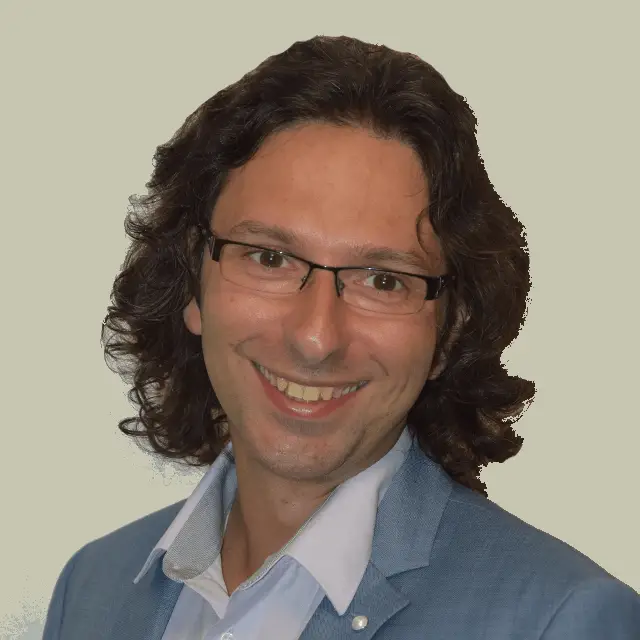
Claudio Sabato is an IT expert with over 15 years of professional experience in Python programming, Linux Systems Administration, Bash programming, and IT Systems Design. He is a professional certified by the Linux Professional Institute.
With a Master’s degree in Computer Science, he has a strong foundation in Software Engineering and a passion for robotics with Raspberry Pi.